Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial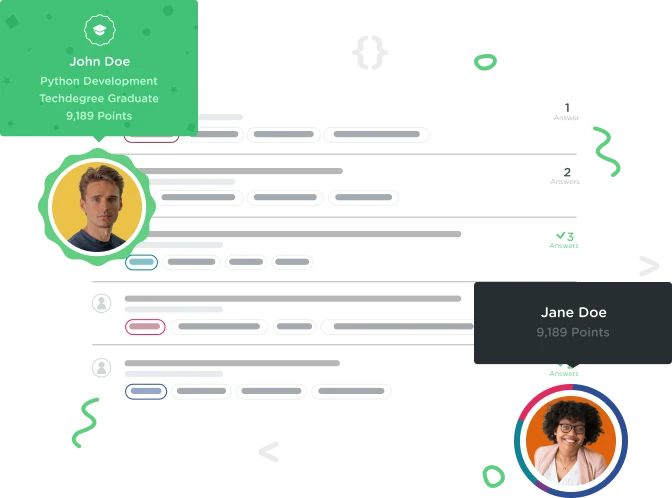

Victor Börjesson
510 Pointsi dont really get where to go from this
I dont understand what to do
namespace Treehouse.CodeChallenges
{
class Polygon
{
public readonly int NumSides;
public Polygon(int numSides)
{
NumSides = numSides;
}
}
class Square : Polygon
{
public readonly int SideLength;
public NumSides(int sideLength) : base(4)
{
}
}
}
2 Answers

andren
28,558 PointsYour solution is quite close, there are only two issues:
You have named your constructor
NumSides
which is incorrect, it should be named the same as the class it is within, which isSquare
.You need to set the
SideLength
field equal to thesideLength
parameter within the constructor, in the same way as is done in thePolygon
constructor.
Like this:
namespace Treehouse.CodeChallenges
{
class Polygon
{
public readonly int NumSides;
public Polygon(int numSides)
{
NumSides = numSides;
}
}
class Square : Polygon
{
public readonly int SideLength;
public Square(int sideLength) : base(4) // Renamed NumSides to Square
{
SideLength = sideLength; // Set SideLength field equal to sideLength parameter.
}
}
}

David Tomko
7,744 PointsJust gotta change the the constructor ('NumSides') to match the Class('Square') and Set SideLength = to the parameter('sideLength')
namespace Treehouse.CodeChallenges
{
class Polygon
{
public readonly int NumSides;
public Polygon(int numSides)
{
NumSides = numSides;
}
}
class Square : Polygon
{
public readonly SideLength;
public Square(int sideLength) : base(4)
{
SideLength = sideLength;
}
}
}