Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial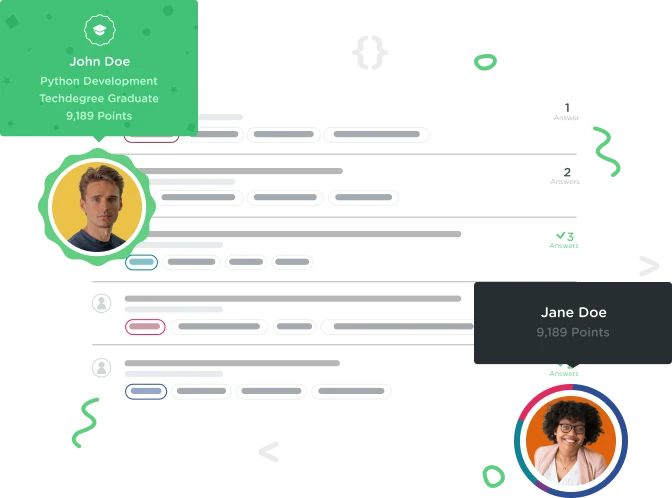

Moreno Halilovic
2,781 PointsI don't see the problem
The program executes just fine when I move it to the VisualStudion and it gives the desired result.
However, the website refuses to accept it and does not throw any compile errors. Only shows a message "Bummer! Try again!".
Am I missing something?
using System;
namespace Treehouse.CodeChallenges
{
class Program
{
static void Main()
{
while (true)
{
try
{
Console.Write("Enter the number of times to print \"Yay!\": ");
string input = Console.ReadLine();
if (input != null)
{
int count = int.Parse(input);
for (int i = 0; i < count; i++)
{
Console.WriteLine("Yay!");
}
break;
}
Console.WriteLine("You must enter a whole number.");
continue;
}
catch (FormatException)
{
Console.WriteLine("You must enter a whole number.");
continue;
}
break;
}
}
}
}
2 Answers
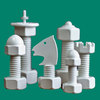
Steven Parker
231,269 PointsThe program the challenge describes should only run once.
You have an outer loop that prevents the program from exiting after it reads a bad input and prints an error message.
This is a great example of why testing challenges externally might not be helpful. If you've misunderstood the instructions, you will also be likely to misinterpret the results.
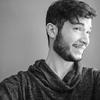
Damian Adams
21,351 PointsTry removing the error handling for the int parsing to see if the challenge will let you through. But you did good in thinking about how to handle error cases.
However, I thought your code could be made more readable and went ahead and made a few modifications, cutting the lines of code in about half while preserving the functionality. Take a look!
using System;
namespace Treehouse.CodeChallenges
{
class Program
{
static void Main()
{
Console.Write("Enter the number of times to print \"Yay!\": ");
int count;
while(!int.TryParse(Console.ReadLine(), out count))
{
Console.Write("You must enter a whole number. Try again: ");
}
for(int i = 0; i < count; i++)
{
Console.WriteLine("Yay!");
}
}
}
}
It's alright if you don't understand a few of the parts, you'll get familiar with the out
keyword and TryParse
method as you move along the Learn C# track.
But it turns out that littering your code with a bunch of continue
and break
statements make your code harder to read, doesn't it? Try to avoid using them as much as possible!