Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial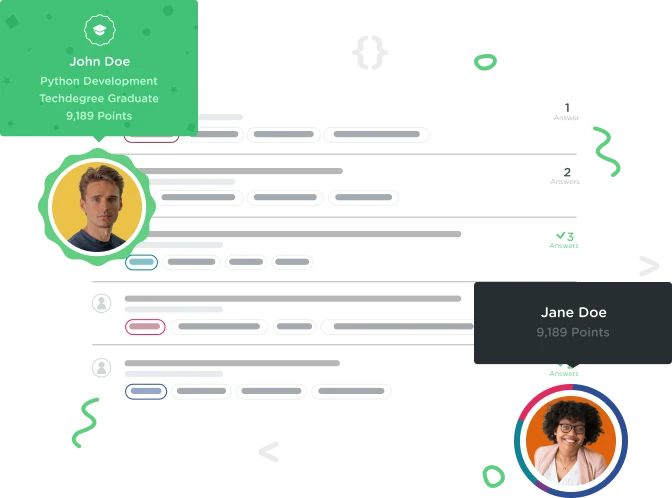
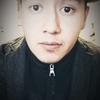
Nelson J
7,411 PointsI don't see what's wrong with my guess the number program.
I don't see why my program never even loads.
// generate random number between 1 and 10
var randomNumber = Math.floor(Math.random() * 10 + 1);
// computer guesses 5
var guessNum = 5;
// counter
var count = 0;
// while computer hasn't guessed the random number, increment the counter by one.
while (guessNum !== randomNumber) {
count += 1;
// If the computer guesses random number, log random number and number of attempts to the console. Otherwise, log the random number and the number the computer guessed.
if(guessNum === randomNumber) {
console.log("random number is " + randomNumber + " and it took " + count + " number of guesses");
}
else {
console.log("random number is " + randomNumber + " and the computer guessed " + guessNum);
}
}
Can you tell me what's wrong with it? Thank you very much.
2 Answers

KRIS NIKOLAISEN
54,971 PointsguessNum should be one of the possible values for randomNumber so inside the loop
guessNum = Math.floor(Math.random() * 10 + 1);

KRIS NIKOLAISEN
54,971 PointsYour guess number never updates inside the loop. So unless the random number generated is 5 you have an infinite loop.
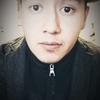
Nelson J
7,411 PointsI have updated the guessNum to be guessNum += 1 inside the loop but it won't work either.
Nelson J
7,411 PointsNelson J
7,411 PointsThank you, that worked and I can see why now. :)