Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial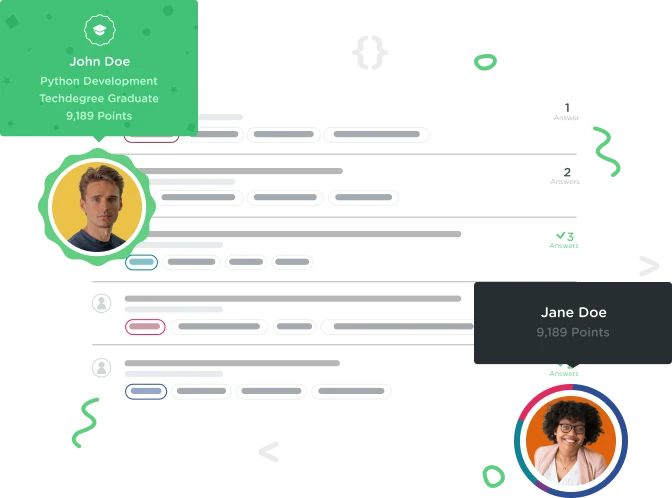
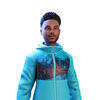
Calvin Secrest
24,815 PointsI don't think I quite grasp multidimensional arrays, and therefore am stuck on the challenge.
I'm still a little confused as about the syntax of the multidimensional arrays. I have this code, but it says the index was out of bounds. Can you explain to me what I'm doing wrong, and what I need to do to fix it without giving the answer away? BTW, here is the link to the challenge in case you need to look at it:
https://teamtreehouse.com/library/c-collections/arrays/multidimensional-arrays
namespace Treehouse.CodeChallenges
{
public static class MathHelpers
{
public static int[,] BuildMultiplicationTable(int maxFactor)
{
var maxFactorPlusOne = maxFactor + 1;
int[,] multiplicationTable = new int[maxFactor, maxFactorPlusOne];
for (int row = 0; row < multiplicationTable.Length; row++)
{
for (int column = 0; column < multiplicationTable[row, maxFactorPlusOne]; column++)
{
multiplicationTable[row, column] = row * column;
}
}
return multiplicationTable;
}
}
}
1 Answer
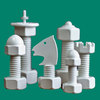
Steven Parker
231,269 Points
You're very close, but you still have a couple of issues:
- When sizing your array, both dimensions need to be maxFactorPlusOne.
- Your row and column loops should also use maxFactorPlusOne as the limit in the condition expression.
Calvin Secrest
24,815 PointsCalvin Secrest
24,815 PointsI change the row and column loops to maxFactorPlusOne and now I'm getting the error:
namespace Treehouse.CodeChallenges { public static class MathHelpers { public static int[,] BuildMultiplicationTable(int maxFactor + 1) { var maxFactorPlusOne = maxFactor + 1; int[,] multiplicationTable = new int[maxFactor, maxFactorPlusOne];
}