Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial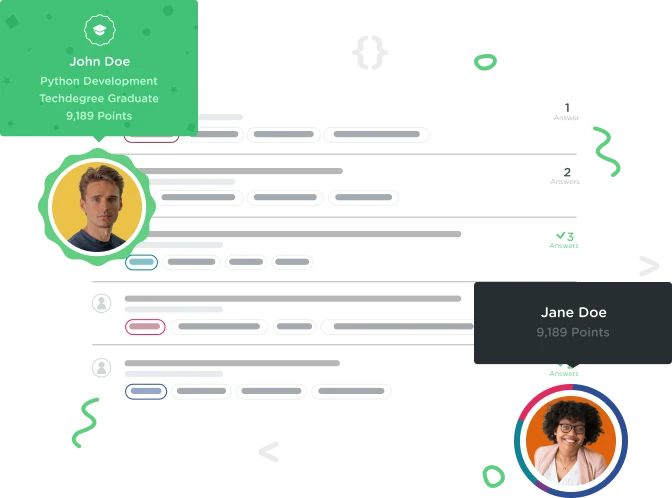

Shafeeq Ahmed
6,058 PointsI don't understand
I don't understand the try, catch blocks and exception class. I am totally confused. I don't really understand this video. Can anyone help me understand?
1 Answer

Maximillian Fox
Courses Plus Student 9,236 PointsYou can use the try-catch block in order to test a piece of code, and if that code fails, handle that error, without stopping the entire script from running. Let's consider the following function both with and without a try catch block.
<?php
// This function will take a number $x and return 1 / $x
function reciprocal($x){
if(!is_int($x)){
// We want to make the function stop as the argument is invalid.
throw new Exception('The argument must be an integer');
}
if($x === 0){
// We want to make the function stop as you can't divide by 0.
throw new Exception('We cannot divide by 0, baaaad things wil happen!');
}
return 1/$x;
}
?>
Now let's call the function without try-catch. Here's what will happen:
<?php
echo reciprocal('crap') . '<br />'; // Code will stop running here.
echo reciprocal(0) . '<br />';
echo reciprocal(4) . '<br />';
?>
This will return Fatal Error: Uncaught Exception: The argument must be an integer ... blah
So now what happens if we wrap just the first function call in a try catch block, like this:
<?php
try{
echo reciprocal('crap') . '<br />'; // This will cause the first exception to be thrown
} catch (Exception $e) { // Just assigning Exception to a variable $e, but you can call it $nooo, etc...
echo $e->getMessage(); // This will return the exception message from the first input.
}
echo reciprocal(0) . '<br />'; // The code will stop running here.
echo reciprocal(4) . '<br />';
?>
This will return: The argument must be an integer Fatal error: Uncaught Exception: We cannot divide by 0, baaaad things wil happen!
So you can see that although the first one failed, it returned the error and thus put out the error message.
So let's use 2 try-catch blocks, like this!
<?php
try{
echo reciprocal('crap') . '<br />'; // This will cause the first exception to be thrown
} catch (Exception $e) { // Just assigning Exception to a variable $e, but you can call it $nooo, etc...
echo $e->getMessage(); // This will return the exception message from the first input.
}
try{
echo reciprocal(0) . '<br />'; // The code will throw the second exception but keep running.
} catch (Exception $e) {
echo $e->getMessage();
}
echo reciprocal(4) . '<br />'; // This will still echo
?>
This will return
The argument must be an integer
We cannot divide by 0, baaaad things wil happen!
0.25
So you can see using try catch blocks can be useful in handling your errors but keep it running.
So, that seems all long winded I bet you're saying. Why not return false instead of wrapping in try-catch? Well, let's do more than maths stuff. :)
Let's try something more realistic. Lets say we want to validate a list of URLs in an array. For simplicity's sake, we will say a URL is valid if it starts with http:// or https://. I will use a regex match for this (but essentially if you don't know preg_match, think of it as just a more powerful strpos function that returns true if the string matches the regex. Check it out on the PHP manual if you are unsure.
<?php
// Checks a list of URLs to see if they are valid, Throws exception on first invalid URL
function checkUrls($url_array){
foreach($url_array as $key => $url){
if(!preg_match('/^https?:\\/\\//', $url)){ //Does the URL have http:// or https:// at the start?
throw new Exception($key); //If not, throw the key of that URL to the exception handler for processing.
}
}
return true;
}
?>
Now let's setup the exception handler. The reason I threw the key to the exception handler is because I wanted it to then unset that array key containing the invalid URL. I can encase that in a while loop, and here's where the magic happens.
<?php
$urls_array = array(
'http://example.com/',
'duff beer',
'https://www.anotherexample.com',
'crappy liiiiink!!!',
'trolololol'
);
$valid = false;
while($valid === false){
try{
$valid = checkUrls($urls_array);
} catch (Exception $e){
unset($urls_array[$e->getMessage()]); //Handler will remove the invalid URL from the array.
}
}
print_r($urls_array);
?>
Now, this will return the $urls_array with ONLY the valid URLs:
Array ( [0] => http://example.com/ [2] => https://www.anotherexample.com )
Test these snippets in workspaces and try for yourself :) Good luck!
Murat Hasdemir
Front End Web Development Techdegree Graduate 20,968 PointsMurat Hasdemir
Front End Web Development Techdegree Graduate 20,968 Pointswell try/catch mostly used on situations that not on your hand like connecting other systems (api services or databases etc.) you basically tell your server to try to connect/perform this action and if connection/perform successful continue but if there is a problem like api services/database down, entered wrong username password for system or the calling method is wrong inform me with $e variable. it will make sense when you start to use it like most of the debuging systems.