Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial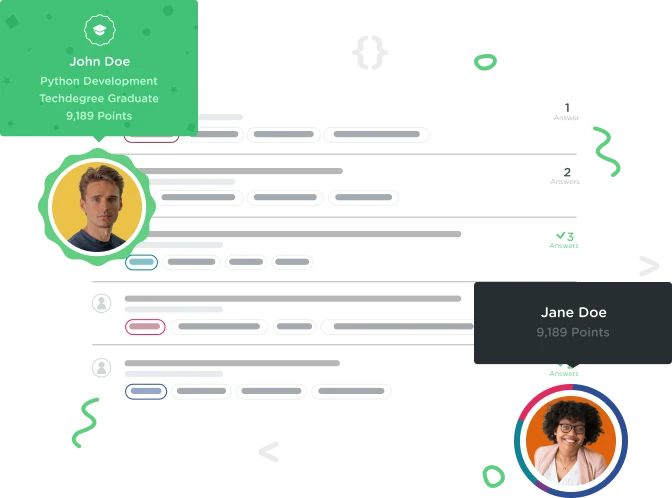

David Daly
2,659 PointsI don't understand closures
help?
/**
For this code challenge, let’s define a math operation as a function that
carries out some work on two integers and returns an integer as well. An
example is the function below, `differenceBetweenNumbers`, which takes two
integers and calculates the difference between the numbers. After calculating,
it returns the difference.
*/
func differenceBetweenNumbers(a: Int, b:Int) -> (Int) {
return a - b
}
// Enter your code below
1 Answer
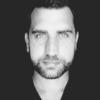
Jhoan Arango
14,575 PointsHello David:
So the challenge wanted us to create a higher order function. The function you have to create is one that takes another function of the same TYPE (from the example function they provided you with), as an argument in its parameter.. What is a function Type? Think of it as like any other type in swift. Int, Bool, Double, String etc.
Let's see what a function type is:
func functionType(a: Int) -> Int {
// Some code
}
// The type of this function is (Int) -> Int
// (Int) -> Int <--- That is the type
Now, that we know what is a function type, let’s go into our challenge code and see the type of the function they provided.
func differenceBetweenNumbers(a: Int, b:Int) -> (Int) {
return a - b
}
// The type of “differenceBetweenNumbers" is "(Int, Int) - > Int".
/*
Now that we have a TYPE, we want to create a function that takes in
this TYPE of function as an argument in its parameter.
*/
// It looks something like this:
func mathOperation(anyAddFunc: (Int, Int) -> Int, a: Int, b: Int) -> Int{
return anyAddFunc(a,b)
}
// Calling the function
var someVariable = mathOperation(differenceBetweenNumbers, a: 10, b: 5)
// This will give you the result of 5
}
Notice how the first parameter for mathOperation is of the same type (Int,Int) -> Int and is named anyAddFunc, the second and third parameters which are named a, and b are of type Int. These are used as the two input values for the provided differenceBetweenNumbers function.
So in conclusion, we created a function that takes in as an argument in its parameter another function of the same type. And at the same time we passed it values so that it can do its calculation.
I suggest reading the book from apple " The Swift Programming Language ", it helps a lot.
Hope that helps, let me know if there something you don’t understand.