Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial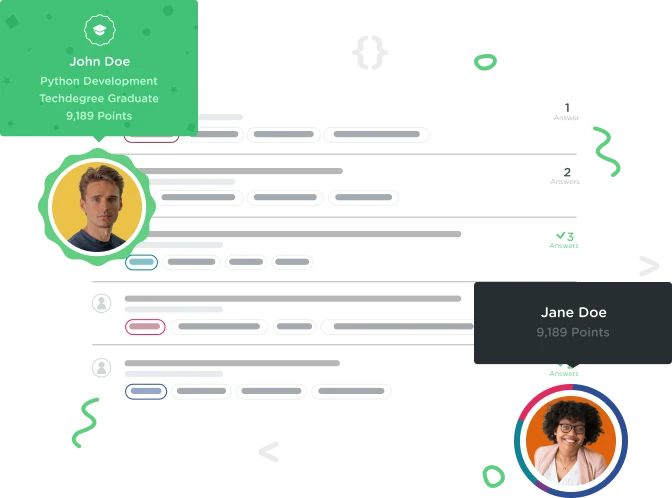
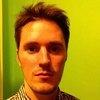
Brendan Whiting
Front End Web Development Techdegree Graduate 84,735 PointsI don't understand "{ get }" and "{ get set }".
protocol AnimalType {
var numberOfLegs: Int { get }
}
protocol PetType: AnimalType {
var cuddlyName: String { get }
}
struct Dog: PetType {
var numberOfLegs: Int
var cuddlyName: String
}
I don't understand what happens when we say the property will have a getter, or a getter and a setter. How is that different from saying it will be a variable or a constant? On this line: var numberOfLegs: Int { get }
, will that property not be able to be set even though it's a variable, not a constant, because we said it wouldn't have a setter method?
2 Answers
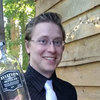
Evan Demaris
64,262 PointsHi Brendan,
Getter-only properties, AKA read-only computed properties, return different results as you change the variables they read from, but the way the variables are handled inside that property can't be changed (or, set). It's like saying that sum = a + b; you wouldn't want someone to be able to change the fact that the property 'sum' will return a + b, but you want to allow them to tell 'sum' what a and b should be.
The Swift documentation on read-only computed properties is pretty good too, if that didn't make sense.

Martin Wildfeuer
Courses Plus Student 11,071 PointsEvan Demaris answer is correct. Let me add my two cents here, a little Playground experiment
protocol TestProtocol {
var testVar: Int { get }
}
class TestClass: TestProtocol {
var testVar: Int = 0
}
// As Swift knows that this is a TestClass,
// we can set it without any errors
let testObject = TestClass()
testObject.testVar = 1
// We could, however, have an array of objects that conform to TestProtocol
// These objects could be of any class, the only thing that we know for sure is
// that the getter for testVar is defined, it could be a regular variable or a
// computed property, or even a public var with a private setter
let testArr: [TestProtocol] = [testObject]
// We know that this is a TestClass and it has a setter, we set it just a few lines before.
// However, an exception will be thrown if we try to set it now, as Swift only knows this object
// conforms to TestProtocol and a setter might not be implemented
var aClassThatConformsToTestProtocol = testArr[0]
aClassThatConformsToTestProtocol.testVar = 10 // Error
// If you change the protocol to
protocol TestProtocol {
var testVar: Int { get set }
}
// it can be set without errors
@Nathan Tallack You got a point here, I think it's all for readability.
Hope that's not too confusing :)
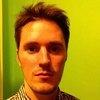
Brendan Whiting
Front End Web Development Techdegree Graduate 84,735 Pointsthank you that helps.
Nathan Tallack
22,159 PointsNathan Tallack
22,159 PointsI was always a little confused about why it is not just { get } or { set }. Isn't the get implied when you have a set?
Brendan Whiting
Front End Web Development Techdegree Graduate 84,735 PointsBrendan Whiting
Front End Web Development Techdegree Graduate 84,735 PointsThank you, that makes sense.