Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial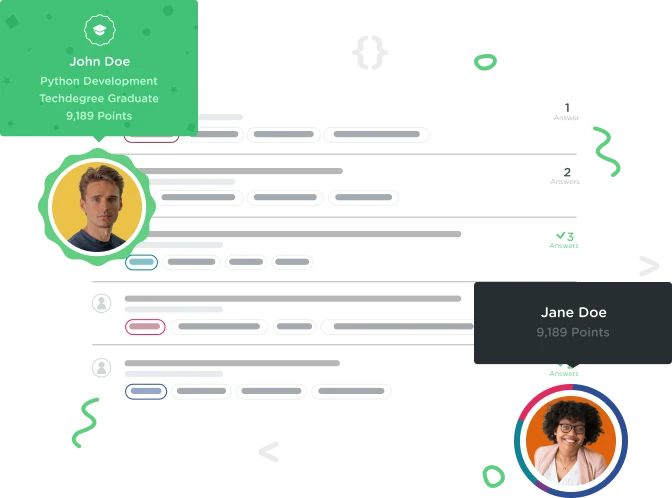

Raul Cisneros
7,319 PointsI don't understand getters and setters.
In most javascript I can see a mental image of how data is getting passed around, but with getters and setters I have hit a brick wall. I have looked at multiple youtube videos and have gone through this course several times and for some reason I cant get an understanding of how getters and setters work. I am to the point, by repetition, that I know where to put a getter and where to put a setter, wether it's in a class, function constructor, or an object. Syntactically I get it, but I don't understand why or how it does what it does. So, the question in task 2 of 2, I don't understand the question. I don't know what it wants. Here is the question... If the student's level is Junior or Senior, the value of the backing property should be equal to the parameter passed to the setter method. If the student is only a Freshman or Sophomore, set the "_major" backing property equal to 'None'.
class Student {
constructor(gpa, credits){
this.gpa = gpa;
this.credits = credits;
}
stringGPA() {
return this.gpa.toString();
}
get level() {
if (this.credits > 90 ) {
return 'Senior';
} else if (this.credits > 60) {
return 'Junior';
} else if (this.credits > 30) {
return 'Sophomore';
} else {
return 'Freshman';
}
}
set major(major) {
this._major = major;
}
}
var student = new Student(3.9, 60);
1 Answer
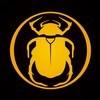
rydavim
18,814 PointsThe goal is to test a condition before setting the student's major
. In this case, you only want to set the major
property to the parameter if the student is a Junior or Senior. If they are a Freshman or Sophomore they don't need to have declared a major yet, and it should be set to None
.
set major(major) {
// Is the student a Junior or Senior?
this._major = major; // Then you can use your major set.
// If they are a Freshman or Sophomore, though...
// They should have a major of None for now.
}
Hopefully that helps you progress, but let me know if you still have any questions. You've got the right idea! Happy coding!
Update:
Okay, so you'll need to use an if
block to check for what level the student is at. If you need to review if ... else
statements, try taking a look at the MDN page.
set major(major) {
// test if the student's (this) level is Junior or (|) Senior
if (this.level == "Junior" | this.level == "Senior") {
this._major = major; // if so...set major to the value passed into your setter above
} else { // if they are not a Junior or Senior
this._major = "None"; // their major should be set to None
}
}
You could also use an if...elif...else
to check against Freshman and Sophomore if you want to have an else
to check for values outside of the four you are expecting.
This is a pretty important challenge concept, so please let me know if you still have questions or don't understand something about this solution. I'll be happy to answer any follow-ups. :)
Raul Cisneros
7,319 PointsRaul Cisneros
7,319 PointsSorry, but that didn't help.
rydavim
18,814 Pointsrydavim
18,814 PointsI've updated my answer with a commented version of one possible solution. Make sure you let me know if you still have any lingering questions about it.