Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial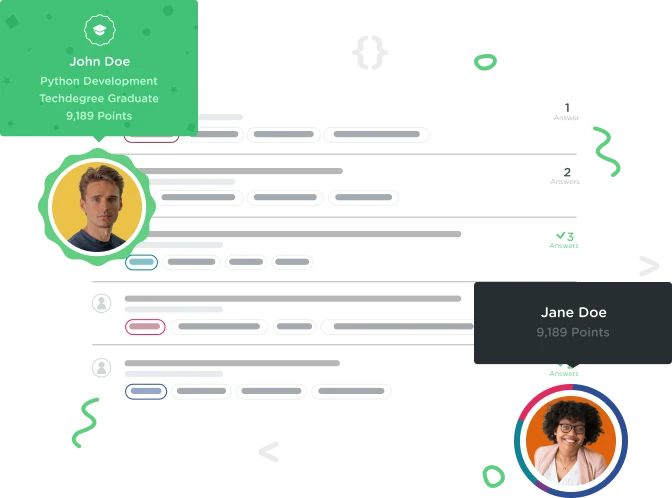
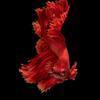
Michael Williams
Courses Plus Student 8,059 PointsI don't understand how my constant is getting assigned to the wrong element (or which element that is).
In the video, we come up with the code below. When you click the toggleList
button, the text in the p.description
element disappears when pressing it to show the list again. The reason, as Guil explains, is that due to the toggleList
button being called first, it results in button
being assigned to the wrong element. Could someone break this down for me so that:
- I understand why this is happening, because I'm just not getting it.
- Which element is it being assigned to?
Thanks!
JavaScript
const toggleList = document.getElementById("toggleList");
const listDiv = document.querySelector(".list");
const input = document.querySelector("input");
const p = document.querySelector("p.description");
const button = document.querySelector("button");
toggleList.addEventListener(("click"), () => {
if (listDiv.style.display === "none") {
toggleList.textContent = "Hide list"
listDiv.style.display = "block";
} else {
toggleList.textContent = "Show list"
listDiv.style.display = "none";
}
});
button.addEventListener("click", () => {
p.innerHTML = input.value + ":"
});
HTML
<!DOCTYPE html>
<html>
<head>
<title>JavaScript and the DOM</title>
<link rel="stylesheet" href="css/style.css">
</head>
<body>
<h1 id="myHeading">JavaScript and the DOM</h1>
<p>Making a web page interactive</p>
<button id="toggleList">Hide list</button>
<div class="list">
<p class="description">Things that are purple:</p>
<input type="text" class="description">
<button class="description">Change list description</button>
<ul>
<li>grapes</li>
<li>amethyst</li>
<li>lavender</li>
<li>plums</li>
</ul>
</div>
<script src="app.js"></script>
</body>
</html>
2 Answers

KRIS NIKOLAISEN
54,968 PointsWith the two buttons querySelector("button") will select the first button element which in this case is the button with id togglelist
const button = document.querySelector("button");
You can see this by logging the id to the console here
button.addEventListener("click", () => {
p.innerHTML = input.value + ":"
console.log(button.id)
You also notice that the toggle list button updates the description.
If however you move the toggle list button below the div in the HTML
</div>
<button id="toggleList">Hide list</button>
<script src="app.js"></script>
both buttons behave as expected because the button with class description is now first. Alternatively you can select the correct button by including the class
const button = document.querySelector("button.description");
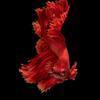
Michael Williams
Courses Plus Student 8,059 PointsThank you, Kris. That was a thorough explanation that cleared things up perfectly. Also, that's a nifty little trick, .id
. I didn't know that was a thing you could do. I'll try that out next time.