Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial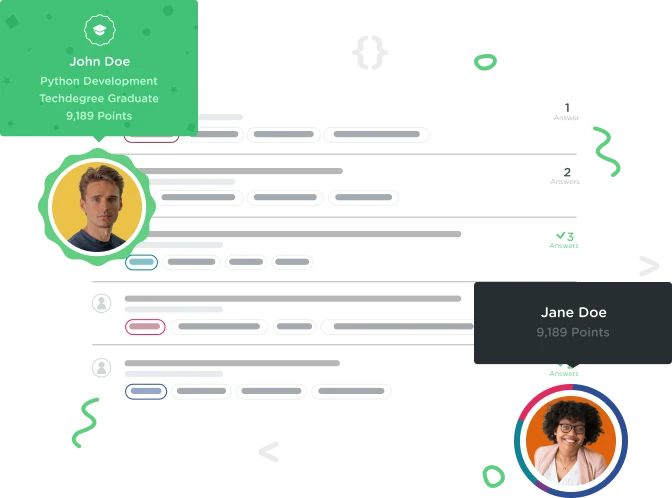
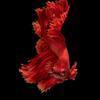
Michael Williams
Courses Plus Student 8,059 PointsI don't understand how the display property being set to an empty string still allows RSVPs to be shown.
I read the MDN, but that didn't help. So here I am. Can someone break down what the empty string value of li.style.display = "";
is doing a little more simplistic than Guil? To me, if it's an empty string, then nothing would be displayed in the DOM except an empty string? ¯_(ツ)_/¯
const form = document.getElementById("registrar");
const input = form.querySelector("input");
const mainDiv = document.querySelector(".main");
const ul = document.getElementById("invitedList");
const div = document.createElement("div");
const filterLabel = document.createElement("label");
const filterCheckBox = document.createElement("input");
filterLabel.textContent = "Hide those who haven't responded";
filterCheckBox.type = "checkbox";
div.appendChild(filterLabel);
div.appendChild(filterCheckBox);
mainDiv.insertBefore(div, ul);
filterCheckBox.addEventListener("change", (e) => {
const isChecked = e.target.checked;
const lis = ul.children;
if (isChecked) {
for (let i = 0; i < lis.length; i += 1){
let li = lis[i];
if (li.className === "responded") {
li.style.display = "";
} else {
li.style.display = "none";
}
}
} else {
for (let i = 0; i < lis.length; i += 1){
let li = lis[i];
li.style.display = "";
}
}
});
function createLI(text) {
const li = document.createElement("li");
const span = document.createElement("span");
span.textContent = text;
li.appendChild(span);
const label = document.createElement("label");
label.textContent - "Confirmed";
const checkbox = document.createElement("input");
checkbox.type = "checkbox";
label.appendChild(checkbox);
li.appendChild(label);
const editButton = document.createElement("button");
editButton.textContent = "edit";
li.appendChild(editButton);
const removeButton = document.createElement("button");
removeButton.textContent = "remove";
li.appendChild(removeButton);
return li;
}
form.addEventListener("submit", (e) => {
e.preventDefault();
const text = input.value;
input.value = "";
const li = createLI(text);
ul.appendChild(li);
});
ul.addEventListener("change", (e) => {
const checkbox = event.target;
checked = checkbox.checked;
const listItem = checkbox.parentNode.parentNode;
if (checked) {
listItem.className = "responded";
} else {
listItem.className = "";
}
});
ul.addEventListener("click", (e) => {
if (e.target.tagName === "BUTTON") {
const button = e.target;
const li = button.parentNode;
const ul = li.parentNode;
if (button.textContent === "remove") {
ul.removeChild(li);
console.log("element has been obliterated!");
} else if (button.textContent === "edit") {
const span = li.firstElementChild;
const input = document.createElement("input");
input.type = "text";
input.value = span.textContent;
li.insertBefore(input, span);
li.removeChild(span);
button.textContent = "save";
} else if (button.textContent === "save") {
const input = li.firstElementChild;
const span = document.createElement("span");
span.textContent = input.value;
li.insertBefore(span, input);
li.removeChild(input);
button.textContent = "edit";
}
}
});
<!DOCTYPE html>
<html>
<head>
<meta name="viewport" content="width=device-width, initial-scale=1">
<title>RSVP App</title>
<link href="https://fonts.googleapis.com/css?family=Courgette" rel="stylesheet">
<link href="https://fonts.googleapis.com/css?family=Lato:400,700" rel="stylesheet">
<link href="css/style.css" rel="stylesheet">
</head>
<body>
<div class="wrapper">
<header>
<h1>RSVP</h1>
<p>A Treehouse App</p>
<form id="registrar">
<input type="text" name="name" placeholder="Invite Someone">
<button type="submit" name="submit" value="submit">Submit</button>
</form>
</header>
<div class="main">
<h2>Invitees</h2>
<ul id="invitedList"></ul>
</div>
</div>
<script type="text/javascript" src="app.js"></script>
</body>
</html>
1 Answer

Adam Kubriczky
4,405 PointsHello,
The display property tells the browser how to show an element, not what to show. Note that, when you manipulate style with javascript, then you do it by modifying the inline style of the element. So when you clear that inline style (e.g. by setting the display property to an empty string), then it is going to use the display setting higher in the CSS hierarchy.