Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial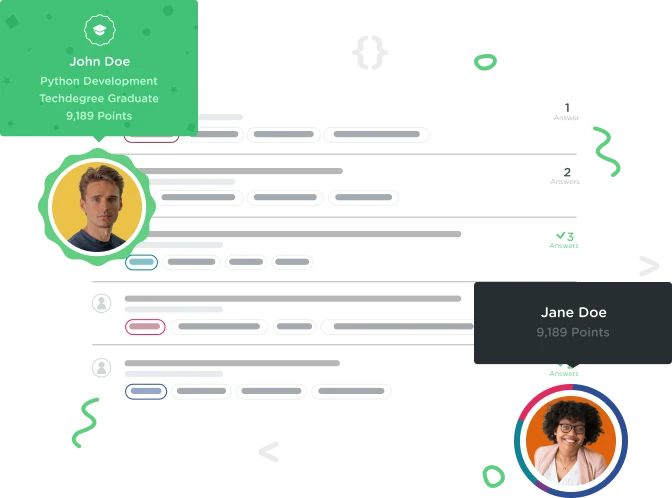

Amy Murphy
6,172 PointsI don't understand how to do this
I know I'm not doing this right, but to be honest I don't know exactly how to do it. Any help would be much appreciated!
import random
class Die:
def __init__(self, sides=2):
if sides < 2:
raise ValueError("Can't have fewer than two sides")
self.sides = sides
self.value = random.randint(1, sides)
def __int__(self):
return self.value
def __add__(self, other):
return int(self) + other
def __radd__(self, other):
return self + other
class D20(Die):
def __init__(self, sides=20):
super().__init__(sides)
from dice import D20
class Hand(list):
@property
def total(self):
return sum(self)
@classmethod
def roll(cls, number_of_dice):
hand = Hand()
for die in number_of_dice:
hand.append(D20(die))
return hand
1 Answer
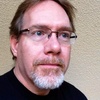
Chris Freeman
Treehouse Moderator 68,457 PointsHey Amy Murphy, youβre very close:
- need to use
hand = cls()
instead ofHand()
since Hand is not yet fully defined - need to use
for die range(number_of_dice):
to repeat based on count. - need to append
D20()
as using D20(die) would append a n-sided die where n is the number_of_die
Not sure why D20
taking an argument passes the challenge. It should have been coded with no sides
parameter and the call to die(20)
fix with 20.
Post back if you need more help. Good luck!!!

Amy Murphy
6,172 PointsThat worked perfectly, thanks for the help!
Amy Murphy
6,172 PointsAmy Murphy
6,172 PointsHere's the question: In the hands.py file import the D20 class from dice.py. Create a classmethod named roll. Since it is a classmethod, it will take cls as an argument. It will also take the number of dice as an argument. Inside the roll classmethod create a new instance of the Hand class and assign it to a variable. Append a D20 to your Hand equal to the number of dice given as an argument to the roll classmethod. Then return the Hand of D20s. For example, if Hand.roll(2) is called, it would return a list with two D20s inside.