Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial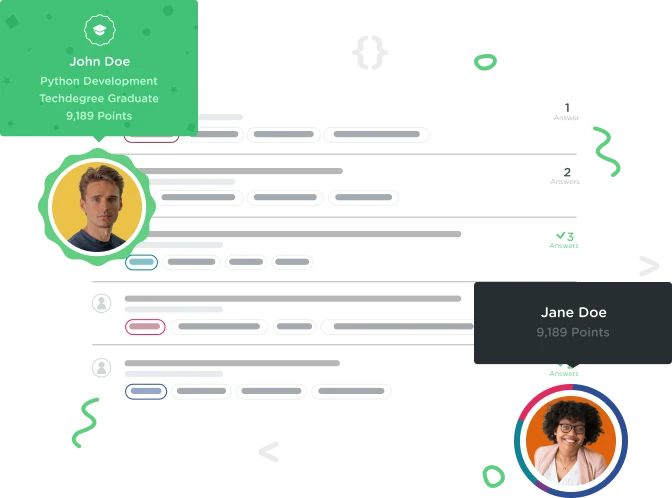

Egi Bhakti Bhakti
1,414 Pointsi dont understand how to use custom initializer?
what is custom initializer? how to use it?
struct RGBColor {
let red: Double
let green: Double
let blue: Double
let alpha: Double
let description: String
// Add your code below
init() {
red = 86.0
green = 191.0
blue = 131.0
alpha = 1.0
description = "red: \(red) green: \(green) blue: \(blue) alpha: \(alpha)"
}
}
1 Answer

noreenqureshipowley
iOS Development Techdegree Student 1,096 PointsHi there,
This question stumped me for 2 days as I was working on it recently. It looks like you were one step ahead of me though because I didn't realize that 'let description: String' was part of the struct because of the spacing. You're also right that init has to have a value for each property of the struct (red, green, blue, alpha, description).
NOTES There are a few things I learned while researching (all notes from Apple Documentation, source mentioned at bottom):
1) Classes and structures must set all of their stored properties to an appropriate initial value by the time an instance of that class or structure is created. Stored properties cannot be left in an indeterminate state.
2) You can set the initial value of a stored property from within an initializer, Alternatively, specify a default property value as part of the property’s declaration. You specify a default property value by assigning an initial value to the property when it is defined.
*This refers to us best and why it is called custom: 3) You can customize the initialization process with input parameters and optional property types, or by assigning constant properties during initialization, as described in the following sections.
3) Structure types automatically receive a memberwise initializer if they do not define any of their own custom initializers. Unlike a default initializer, the structure receives a memberwise initializer even if it has stored properties that do not have default values.
4) When a parameter name for an instance method has the same name as a property of that instance, the parameter name takes precedence, and it becomes necessary to refer to the property in a more qualified way. You use the self property to distinguish between the parameter name and the property name.
ANSWER Taking those notes into account, my code is below. Where our code differs is that I have set the custom initializer's stored values to an initial value of empty constants waiting to be 'instantiated'. The reason I didn't assign the given values (86.0, etc.) was because I wanted a user to be able to change them without having to rewrite code in the initializer. This happens outside the scope of the struct. To do so I used note #4 and specifically set the initializer's parameters and used the self method to identify the struct's properties. Outside the struct you can see I've used a variable colorCode to capture ONLY red, green, blue and alpha. This is the big distinction from using the custom initializer and the memberwise initializer.
~~*CUSTOM INITIALIZER~*~
struct RGBColor {
let red: Double
let green: Double
let blue: Double
let alpha: Double
let description: String
init(red: Double, green: Double, blue: Double, alpha: Double) {
self.red = red
self.green = green
self.blue = blue
self.alpha = alpha
description = "red: \(red), green: \(green), blue: \(blue), alpha: \(alpha)"
}
}
var colorCode = RGBColor(red: 86.0, green: 191.0, blue: 131.0, alpha: 1.0)
print(colorCode)
This returns RGBColor(red: 86.0, green: 191.0, blue: 131.0, alpha: 1.0, description: "red: 86.0, green: 191.0, blue: 131.0, alpha: 1.0")
Here's the same code compared to using a memberwise initializer:
~~*MEMBERWISE INITIALIZER~*~
struct RGB2Color {
let red: Double
let green: Double
let blue: Double
let alpha: Double
let description: String
}
let colorCode2 = RGB2Color(red: 1.0, green: 2.0, blue: 3.0, alpha: 4.0, description: "monkeys")
print(colorCode2)
This returns RGB2Color(red: 1.0, green: 2.0, blue: 3.0, alpha: 4.0, description: "monkeys")
I hope this helps! Good luck!
Here are my sources:
Similar posts:
https://teamtreehouse.com/community/rgb-initializer https://teamtreehouse.com/community/need-help-custom-initialisers-code-challenge-object-oriented-swift-20 https://teamtreehouse.com/community/objectiveoriented-swift-20-custom-initializers
noreenqureshipowley
iOS Development Techdegree Student 1,096 Pointsnoreenqureshipowley
iOS Development Techdegree Student 1,096 PointsHi there,
This question stumped me for 2 days as I was working on it recently. It looks like you were one step ahead of me though because I didn't realize that 'let description: String' was part of the struct because of the spacing. You're also right that init has to have a value for each property of the struct (red, green, blue, alpha, description).
NOTES There are a few things I learned while researching (all notes from Apple Documentation, source mentioned at bottom):
1) Classes and structures must set all of their stored properties to an appropriate initial value by the time an instance of that class or structure is created. Stored properties cannot be left in an indeterminate state.
2) You can set the initial value of a stored property from within an initializer, Alternatively, specify a default property value as part of the property’s declaration. You specify a default property value by assigning an initial value to the property when it is defined.
*This refers to us best and why it is called custom: 3) You can customize the initialization process with input parameters and optional property types, or by assigning constant properties during initialization, as described in the following sections.
3) Structure types automatically receive a memberwise initializer if they do not define any of their own custom initializers. Unlike a default initializer, the structure receives a memberwise initializer even if it has stored properties that do not have default values.
4) When a parameter name for an instance method has the same name as a property of that instance, the parameter name takes precedence, and it becomes necessary to refer to the property in a more qualified way. You use the self property to distinguish between the parameter name and the property name.
ANSWER Taking those notes into account, my code is below. Where our code differs is that I have set the custom initializer's stored values to an initial value of empty constants waiting to be 'instantiated'. The reason I didn't assign the given values (86.0, etc.) was because I wanted a user to be able to change them without having to rewrite code in the initializer. This happens outside the scope of the struct. To do so I used note #4 and specifically set the initializer's parameters and used the self method to identify the struct's properties. Outside the struct you can see I've used a variable colorCode to capture ONLY red, green, blue and alpha. This is the big distinction from using the custom initializer and the memberwise initializer.
~~*CUSTOM INITIALIZER~*~
This returns RGBColor(red: 86.0, green: 191.0, blue: 131.0, alpha: 1.0, description: "red: 86.0, green: 191.0, blue: 131.0, alpha: 1.0")
Here's the same code compared to using a memberwise initializer:
~~*MEMBERWISE INITIALIZER~*~
This returns RGB2Color(red: 1.0, green: 2.0, blue: 3.0, alpha: 4.0, description: "monkeys")
I hope this helps! Good luck!
Here are my sources:
https://developer.apple.com/library/ios/documentation/Swift/Conceptual/Swift_Programming_Language/ClassesAndStructures.html
https://developer.apple.com/library/ios/documentation/Swift/Conceptual/Swift_Programming_Language/Initialization.html#//apple_ref/doc/uid/TP40014097-CH18-ID203
https://developer.apple.com/library/ios/documentation/Swift/Conceptual/Swift_Programming_Language/Methods.html
Similar posts:
https://teamtreehouse.com/community/rgb-initializer https://teamtreehouse.com/community/need-help-custom-initialisers-code-challenge-object-oriented-swift-20 https://teamtreehouse.com/community/objectiveoriented-swift-20-custom-initializers