Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial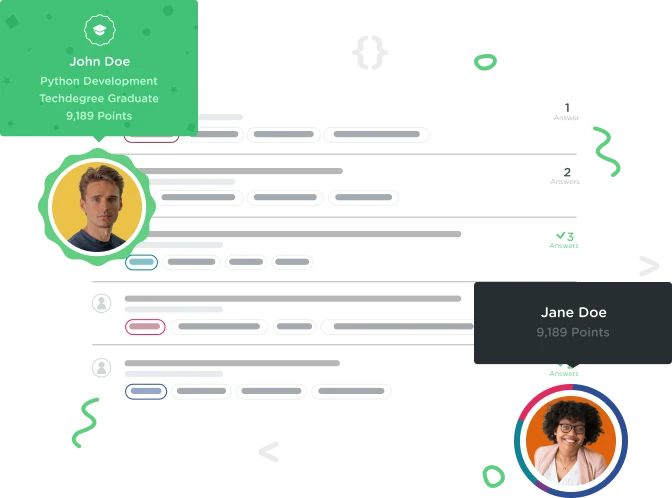
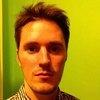
Brendan Whiting
Front End Web Development Techdegree Graduate 84,736 PointsI don't understand "let redValue, let greenValue..." here
Full code:
import UIKit
enum ColorComponents {
case RGB(red: CGFloat, green: CGFloat, blue: CGFloat, alpha: CGFloat)
case HSB(hue: CGFloat, saturation: CGFloat, brightness: CGFloat, alpha: CGFloat)
func color() -> UIColor {
switch self {
case .RGB(let redValue, let greenValue, let blueValue, let alphaValue):
return UIColor(red: redValue/255.0, green: greenValue/255.0, blue: blueValue/255.0, alpha: alphaValue)
case .HSB(let hueValue, let saturationValue, let brightnessValue, let alphaValue):
return UIColor(hue: hueValue/360.0, saturation: saturationValue/100.0, brightness: brightnessValue/100.0, alpha: alphaValue)
}
}
}
ColorComponents.RGB(red: 61.0, green: 120.0, blue: 198.0, alpha: 1.0).color()
I don't understand this line:
case .RGB(let redValue, let greenValue, let blueValue, let alphaValue):
What's happening here? Are we specifying parameter names? Why do we need to use "let"?
1 Answer
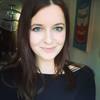
Marina Alenskaja
9,320 PointsHi Brendan
As I understand it, the constants just represent a given value of RGB (red, green and blue + alpha). So like I commented in the below code, we could have written any actual value, but since we are writing a method that has to be flexible to all colors, we use constants to store the values. (Not sure if that's the "correct" explanation, but that's just how I understand it..)
func color() -> UIColor {
switch self {
case .RGB(
let redValue,
let greenValue,
let blueValue,
let alphaValue): return UIColor(
red: redValue/255.0, //this is the same as: red: 234/255.0
green: greenValue/255.0, //this is the same as: green: 62/255.0
blue: blueValue/255.0, //this is the same as: blue: 23/255.0
alpha: alphaValue //this is the same as: alpha: 1.0
)
case .HSB(
let hueValue,
let saturationValue,
let brightnessValue,
let alphaValue): return UIColor(
hue: hueValue/360.0,
saturation: saturationValue/100.0,
brightness: brightnessValue/100.0,
alpha: alphaValue
)
}
}
}
Brendan Whiting
Front End Web Development Techdegree Graduate 84,736 PointsBrendan Whiting
Front End Web Development Techdegree Graduate 84,736 PointsSo are they like parameter names?
Marina Alenskaja
9,320 PointsMarina Alenskaja
9,320 PointsI'm pretty sure they are temporary stored properties - Pasan mentions this in some videos.
Stephen Whitfield
16,771 PointsStephen Whitfield
16,771 PointsHmmm.. Close but not exactly. The constant names are more or less for convenience and readability (most of all readability). Stuff like this becomes more and more essential the more developers you have touching the same codebase as you. The more readable the code, the more maintainable it is. But most importantly, in this case, we're passing those constants to the UIColor init method in our switch statement. Pasan is basically showing you how flexible/powerful enums are.