Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial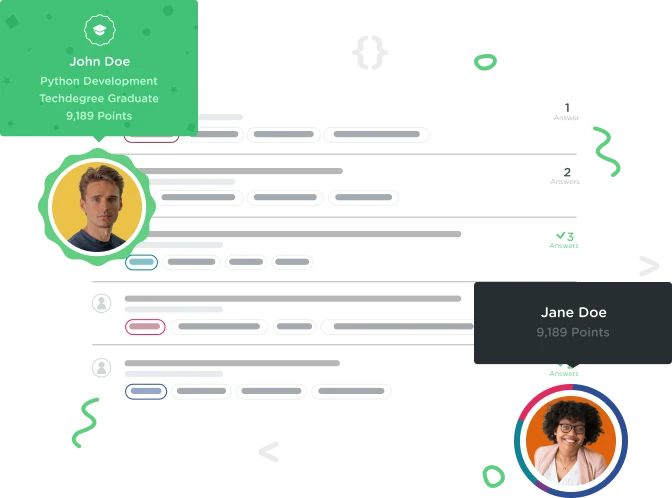

nfs
35,526 PointsI don't understand one thing in this video... That's all.
'use strict';
let teachers = [
{ name: 'Ken', comments: 'Amazing', rating: 4 },
{ name: 'James', comments: 'Astounding', rating: 3 },
{ name: 'Dave', comments: 'Astonishing', rating: 3 },
{ name: 'John', comments: 'Accelerating', rating: 2 },
{ name: 'Andrew', comments: 'Arm-chair-ing', rating: 3 },
{ name: 'Elizabeth', comments: 'Accepting', rating: 5 },
{ name: 'Nick', comments: 'Automating', rating: 6 },
{ name: 'Sarah', comments: 'Amplifying', rating: 7 },
{ name: 'Alena', comments: 'Appending', rating: 8 }
];
teachers.forEach(teacher => {
if (teacher.name === 'Nick') {
console.log(teacher.rating);
}
});
My question is :
What is this teacher parameter used for in the callback function? I don't get it.
Any help is appreciated. Thank you.
4 Answers

nico dev
20,364 PointsHi Nafis Fuad ,
// I think this is what you refer to:
teachers.forEach(teacher => {
if (teacher.name === 'Nick') {
console.log(teacher.rating);
}
});
// Let's see it part by part:
teachers.forEach // -> We will loop through each index in the teachers array
// Now let's put a name to each index of the array, so we can identify them on each cycle of the loop:
// Let's call it teacher (could have been moustachio, or pineapple, and it would work the same.
(teacher => {
// If the name property/key on each index (which happens to be an object) is Nick (it has the value 'Nick')...
if (teacher.name === 'Nick')
//... then print the value of the property/key named 'rating'
console.log(teacher.rating);
//NOTE:
// This:
teacher => {
if (teacher.name === 'Nick') {
console.log(teacher.rating);
}
};
// is the same than:
function (teacher) {
if (teacher.name === 'Nick') {
console.log(teacher.rating);
}
}
Hope that helps, but otherwise feel free to follow up here.
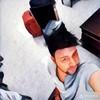
Ari Misha
19,323 PointsHiya there! Lets read it this way. Keep in mind that Teachers array have bunch of objects at each index. Here it goes. For each Teacher ( Teacher represents the current object in the array ), if the current teacher's name is "Nick" the log out the rating for the same teacher. See its all english. Now lets convert this in code:
// forEach teacher in Teachers
teachers.forEach(teacher => {
//if teacher's name is Nick
if (teacher.name === 'Nick') {
//log out the ratings for same teacher in the console
console.log(teacher.rating);
}
});
I hope it helped!
~ Ari

nfs
35,526 PointsAwesome, Nico Trivelli. Thank you

nfs
35,526 PointsThank you, Ari Misha.