Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial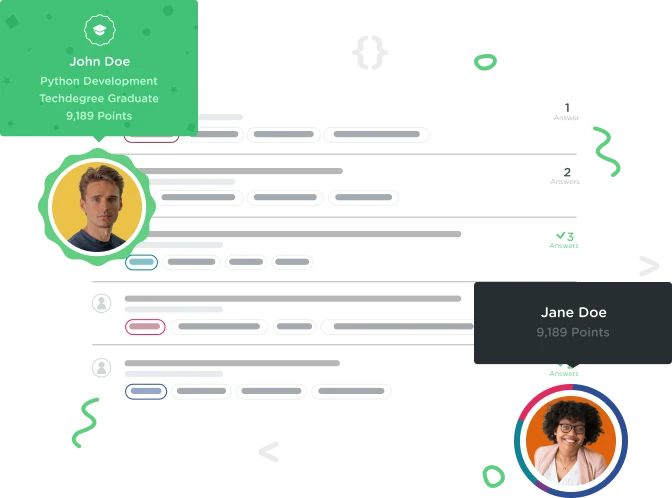
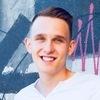
Gene Bogdanovich
14,618 PointsI don't understand overloaded method
We have a method in our Point class:
public int DistanceTo(int x, int y)
{
int xDiff = X - x;
int yDiff = Y - y;
int xDiffSquared = xDiff * xDiff;
int yDiffSquared = yDiff * yDiff;
return (int)Math.Sqrt(xDiffSquared + yDiffSquared);
}
And then we overload this method:
public int DistanceTo(Point point)
{
return point.DistanceTo(point.X, point.Y);
}
And to this method we pass in an instance of foreign point. In the body of that method it is confusing for me to understand when we refer to the point that is the instance of the class and when refer to the other (foreign to that instance) point. Can you please explain in detail? Also it is worth mentioning that apparently there is something wrong with that method because when I do this in the Game class:
Map map = new Map(8, 5);
Point point1 = new Point(1, 1);
Point point2 = new Point(5, 3);
Console.WriteLine("The distance between point 1 and point 2: {0}", point1.DistanceTo(point2));
I get the following output in my console: The distance between point 1 and point 2: 0
And if I pass in literals everything works fine:
Console.WriteLine("The distance between point 1 and point 2: {0}", point1.DistanceTo(5, 3));
The distance between point 1 and point 2: 4
Seems to me that overloaded method finds the distance between the same point.
I would greatly appreciate any help!
1 Answer

Marek Hůlka
5,424 PointsHi,
question no. 1: If you are pointing to foreign instance of variable (or property, method) - meaning in different object - it is alwas prepended by object name (not class name) in this case ex.: point.X (point is instance of Point class in different object and you are pointing to X property of that object). If you want to point to variable (or property, method) in current object there can be nothing prepended ex. X or for better reading you can prepend this ex. this.X (meaning X of this object). This can be used only for nonstatic members. For static members you need to prepend CLASS name (not object name)
question no. 2: Your code is incorrect, that is why is result always 0.
Your code is similar to this:
public int DistanceTo(Point point2)
{
return point2.DistanceTo(point2.X, point2.Y);
}
and it's meaning is - I have some point and I call it's method DistanceTo and I give another point (let's call it point2) to it. And from that metod I'm calling method DistanceTo of point2 and try to compare with object point2 itself. That is why is answer always 0 because point2 distance to itself is zero.
Correctly your method should be like this:
public int DistanceTo(Point point2)
{
return this.DistanceTo(point2.X, point2.Y);
}
or inverted like this:
public int DistanceTo(Point point2)
{
return point2.DistanceTo(this.X, this.Y);
}
and it's meaning: I have object point1 and it's method DistanceTo. I give it another object named point2 and now I'm calling method of point1 to properties of point2 (or inverted call method of point2 on properties of point1).
Sorry for my english. :) If it's not understandable for you I will try rewrite it. :)
Best regards
Marek