Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial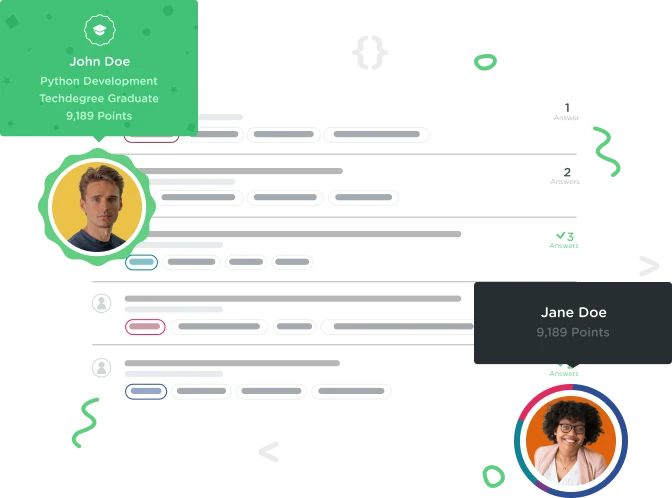
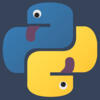
Embie Wong
3,180 PointsI don't understand some parts
Hi, I understand how to convert multidimensional arrays to objects, but I'm a bit confused with changing the for loop. In the video, the for loop looks like this:
for (let i = 0; i < questions.length; i++) {
let question = questions[i].question;
let answer = question[i].answer;
}
Since the questions array was converted into an object, doesn't it now have an index length of one? If so, that means the loop will only run once. Does the questions[i].question code run all the objects in the array?
1 Answer
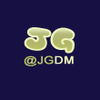
Jonathan Grieve
Treehouse Moderator 91,253 PointsThe loops doesn't change the length, (that is the number of items in the data)
To break it down, questions
refers to the variable that stores the object, which is why we have length as the property in the loop expression.
for (let i = 0; i < questions.length; i++) {
}
And inside we're looping through the key-value pairs of the object where question
and answer
are properties of the object.
for (let i = 0; i < questions.length; i++) {
let question = questions[i].question;
let answer = questions[i].answer;
}
There's no messing around with the actual values in the property. All we're doing here is iterating through them so the values can be displayed. :-)
Gareth Moore
7,724 PointsGareth Moore
7,724 PointsThe
questions
array was not converted into an object. Thequestions
array is still an array. The values inside thequestions
array are now objects. Thus, the loop operates as normal because it is still an array.See below, pay attention to [] which indicates arrays, and {} which indicates objects.