Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial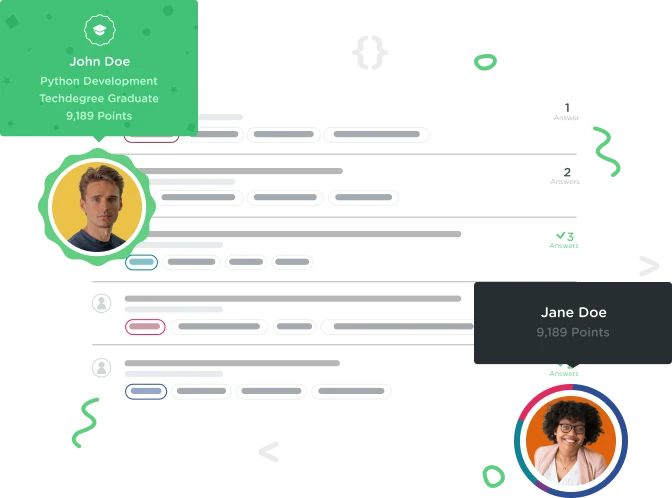

Tan Yang
13,163 PointsI don't understand the Bummer in call center challenge!
why does it prompt that remember to call assist() method, I have already called this method!
import java.util.ArrayDeque;
import java.util.Queue;
public class CallCenter {
Queue<CustomerSupportRep> mSupportReps;
public CallCenter(Queue<CustomerSupportRep> queue) {
mSupportReps = queue;
}
public void acceptCustomer(Customer customer) {
CustomerSupportRep csr = new CustomerSupportRep("Daniel");
/********************************************
* TODO (1)
* Wait until there is an available rep in the queue.
* While there is not one available, playHoldMusic
* HINT: That while assignmentcheck loop syntax we used to
* read files seems pretty similar
********************************************
*/
while(mSupportReps.poll() == null){
playHoldMusic();
}
/********************************************
* TODO (2)
* After we have assigned the rep, call the
* assist method and pass in the customer
********************************************
*/
csr = mSupportReps.poll();
csr.assist(customer);
/********************************************
* TODO (3)
* Since the customer support rep is done with
* assisting, put them back into the queue.
********************************************
*/
mSupportReps.add(csr);
}
public void playHoldMusic() {
System.out.println("Smooooooth Operator.....");
}
}
import java.util.List;
import java.util.ArrayList;
public class CustomerSupportRep {
private String mName;
private List<Customer> mAssistedCustomers;
public CustomerSupportRep(String name) {
mName = name;
mAssistedCustomers = new ArrayList<Customer>();
}
public void assist(Customer customer) {
System.out.printf("Hello %s, my name is %s, how can I assist you.%n",
customer.getName(),
mName);
System.out.println("...");
System.out.println("Is there anything else I can help you with?");
mAssistedCustomers.add(customer);
}
public List<Customer> getAssistedCustomers() {
return mAssistedCustomers;
}
}
public class Customer {
private String mName;
public Customer(String name) {
mName = name;
}
public String getName() {
return mName;
}
}
1 Answer

Yanuar Prakoso
15,196 PointsHi Tan
You need to do some revision in your code, Please pay attention to the notes that I gave to your original code here :
import java.util.ArrayDeque;
import java.util.Queue;
public class CallCenter {
Queue<CustomerSupportRep> mSupportReps;
public CallCenter(Queue<CustomerSupportRep> queue) {
mSupportReps = queue;
}
public void acceptCustomer(Customer customer) {
/************************************************************************************
*You cannot do this you must take csr from the queue called mSupportRep
* not creating new CustomerSupportRep like what you did here:
* CustomerSupportRep csr = new CustomerSupportRep("Daniel");
* you must keep this line unchanged from the start of the challenge:
*************************************************************************************
*/
CustomerSupportRep csr;
/********************************************
* TODO (1)
* Wait until there is an available rep in the queue.
* While there is not one available, playHoldMusic
* HINT: That while assignmentcheck loop syntax we used to
* read files seems pretty similar
********************************************
*/
/*****************************************************************************
* Please pay more attention to the HINT in the TODO (1)
* You need to do one more thing other than what you've done in your while
* loop here:
while(mSupportReps.poll() == null){
playHoldMusic();
}
* That one thing is what will make your while loop becomes assignment check loop
*****************************************************************************
this is how I will code it:*/
while((csr=mSupportReps.poll())==null){
playHoldMusic();
}
// Please note the csr=mSupportReps.poll() inside the while declaration
// There I assigned the csr by poll one CustomerService object from the
// mSupportReps queue and then test it if the value is null.
/********************************************
* TODO (2)
* After we have assigned the rep, call the
* assist method and pass in the customer
********************************************
*/
// csr = mSupportReps.poll();<--- you do not need this if you use assignment check above
// you can straight to this code:
csr.assist(customer);
/********************************************
* TODO (3)
* Since the customer support rep is done with
* assisting, put them back into the queue.
********************************************
*/
//There is nothing wrong here, good job putting a csr back to the mSupportReps queue:
mSupportReps.add(csr);
}
public void playHoldMusic() {
System.out.println("Smooooooth Operator.....");
}
}
I wish this can help a little. Happy coding