Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial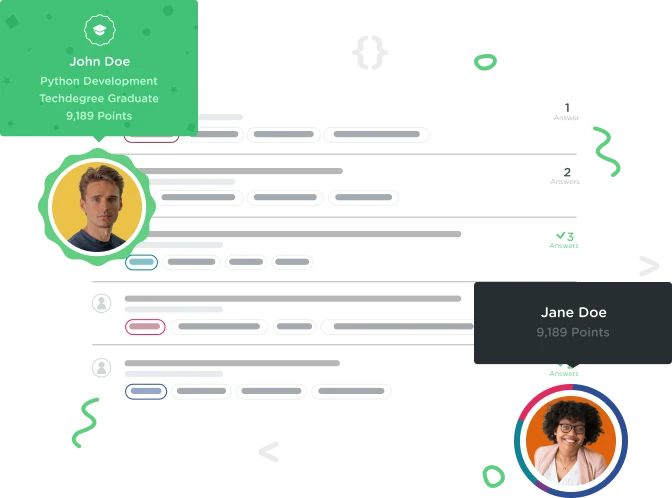

Lukas Johmann
911 PointsI don't understand the challenge
Hello Treehousers, I'm very confused and don't understand functions and lists enough to complete this challenge. Can someone explain to me the steps to take to coplete this challenge?
Challenge Task 1 of 1 OK, I need you to finish writing a function for me. The function disemvowel takes a single word as a parameter and then returns that word at the end.
I need you to make it so, inside of the function, all of the vowels ("a", "e", "i", "o", and "u") are removed from the word. Solve this however you want, it's totally up to you!
Oh, be sure to look for both uppercase and lowercase vowels!
def disemvowel(word):
vowel = "a","e", "o", "u"
for vowel in word:
del(vowel)
return word
2 Answers

Michal Kozák
1,139 PointsHey Lukas,
Your code is kinda on the right path, but I'll give you just some hints, so you can try to figure it out for yourself.
What are you doing in this exercise is, that you're effectively comparing values in two lists, and then not using letters, that are in both of those lists.
- Make your vowels a list using []; you also forgot "i" in there.
- Make a list out of the word argument using list(word) function, like test_word = list(word)
- Create an empty list for storing the non-vowel letters, like new_word = []
- Run for loop for each letter in the test_word, and test in each loop using if statement, whenever the letter is not in the vowels list. If it's not, append that letter to that empty new_word list
- Make a string out of the new_word list using "".join(new_word)
You also need to handle uppercases and lowercases.
I did it maybe lazily, by going like this:
However, much better would be uppercasing or lowercasing each letter in the if statement, using .upper() or .lower() function.
vowels = ["a", "e", "i", "o", "u", "A", "E", "I", "O", "U"]
Good luck!

Lukas Johmann
911 PointsHello Michal! Thanks for your answer, I tried going thru the steps you recommended. I am getting a syntax error on line 9. Can you explain what is wrong with my code?
vowel = ["a", "e", "i", "o", "u"]
test_word = list(word)
new_word = []
for vowel.lower() in test_word:
if letter.lower() not in vowel:
letter += new_word
word.join(new_word)
def disemvowel(word)
return word

Michal Kozák
1,139 PointsHey Lukas!
Good going, you almost got it. This should be your code, right?
def disemvowel(word)
vowel = ["a", "e", "i", "o", "u"]
test_word = list(word)
new_word = []
for vowel.lower() in test_word:
if letter.lower() not in vowel:
letter += new_word
word.join(new_word)
return word
- In your for loop, use simply letter instead of vowel.lower(), because in this step, you're simply telling the loop to stop on "each thing in the thing". This is the place, where the letter variable is created, and which you've correctly used in the rest of the function.
- In your if code, you got the list appending backwards. You have a new_word list, and you just want to add the letter to it, so simply switch the variables, so it goes new_word += letter.
- Lastly, you want to assign list converted to string to a word variable. .join() function requires from you, to put in a character, with which you want the list to be joined. If you simply want all characters together, you do empty quotes like "".join(new_word). You can assign this to the word variable, or you could simply return it right away, like return "".join(new_word)
At the end, you should end up with a code like this:
def disemvowel(word):
vowel = ["a", "e", "i", "o", "u"]
test_word = list(word)
new_word = []
for letter in test_word:
if letter.lower() not in vowel:
new_word += letter
return "".join(new_word)
Hope this works for you, it worked for me in my interpreter.