Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial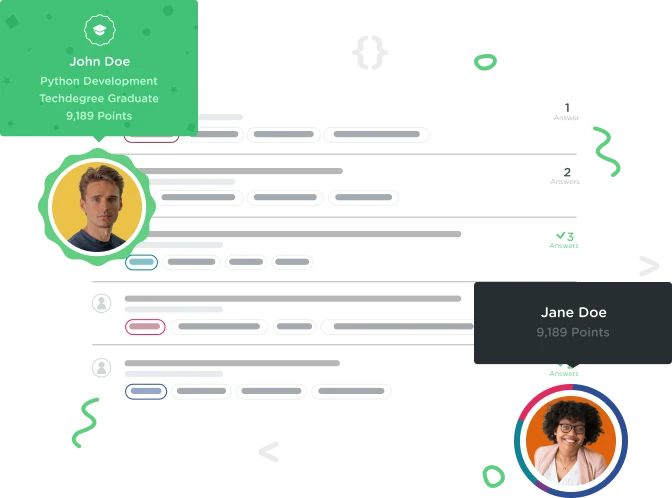

alexis potvin
Front End Web Development Techdegree Student 927 PointsI don't understand the difference between Static and instance...
Everything is in the question please give me exemple so i can understand it good
1 Answer
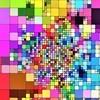
james south
Front End Web Development Techdegree Graduate 33,271 Pointsstatic belongs to the class, non-static belongs to instances. if the class was bank accounts, a static field might be something like the bank's routing number. it is the same for all accounts and no account has any need to change it. there would be only one. the instances of the bank account class would be individual bank accounts, so an instance or non-static field might be the individual account numbers. each account has its own and they are different for each instance. here is a good link for more:
Purvi Agrawal
7,960 PointsPurvi Agrawal
7,960 PointsHi Alexis,
First of all, apologies for late response. Static can be quite intimidating at first but the more you use them, the more you understand.
Instance or objects as the name suggests are the instances of the class. Meaning they represent the properties and methods of a class. Lets say I have a class A and few properties and methods defined in the class. If I declare an instance of a class in another class B, I can use the properties and methods of class A in class B using the instance of class A. The only catch is the properties and methods of class A needs to be public.
public class A{ public int x; public int y;
}
public class B{
A object1 = new A(); // Instance of class A object1.x = 5; // Initializing the property x of class A in class B using the instance of class A
} Whereas, if the class, property or method is declared as static, I can use them without instantiating an object for the class.
class TestA { public static int var1; // Static Member public int var2; // Non-Static Member }
class TestB { TestA.var1 = 6 ; // Success TestA.var2 = 7; // Error as var2 is non-static. }
Important thing to remeber with Statics is that they retain one copy throughtout. So if,
class TestB{ TestA.var1 = 6 ;
public function initial() { TestA.var1 ++; }
public function second() { Console.WriteLine(TestA.var1); // It will output 7; } }
I hope the explain was easy enough to understand. Plz use the msdn link for a better idea : https://msdn.microsoft.com/en-us/library/98f28cdx.aspx