Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial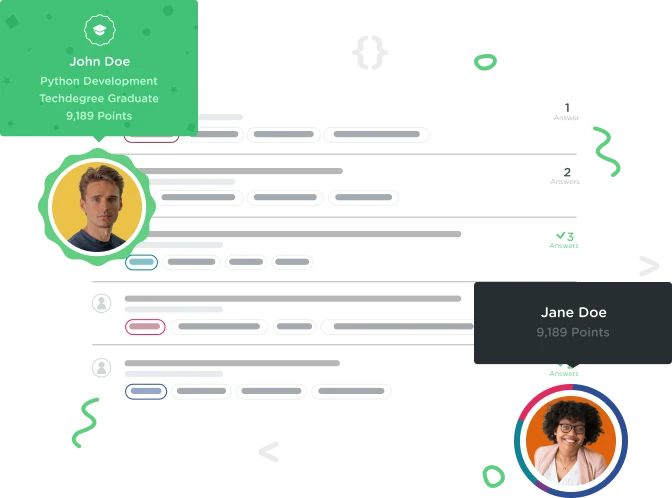

Michael North
2,561 PointsI don't understand the difference between static and instance
I know there is another question out there about static and instance, but to me the answer was not clear. Can someone please give me a detailed explanation on the differences. Thank you!
2 Answers
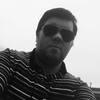
Stephen Kilgore
Java Web Development Techdegree Student 2,610 Pointsa static method is one that is defined directly to the class itself and does not need you to create an instance (or object) of that class to use the method.
If you need to be able to use a certain functionality from one of your classes even when you aren't using it directly with an instance you created, then it needs to be static.
Lets say I make the following class:
public class Car
{
public void drive()
{
//do something to make a car go
}
}
Obviously, that method shouldn't be static because it needs to be applied on Car instance itself.
If, however, I want to directly be able to use a method from the car Class even if I don't have an object, I can declare a static method. Let's say I want to have a method on the Car class that calculates how many miles per minute is in one mile per hour. (I don't know why you'd ever need to do this, but it's the first example that came to mind)
public class Car
{
public void drive()
{
//do something to make a car go
}
public static int milesPerMinute(int milesPerHour)
{
return milesPerHour * 60;
}
}
to use the drive() method that is not static, I need an object:
Car myCar = new Car();
Then, I can access myCar.drive();
drive() will NOT work without an instance to use it on.
If for some reason I just want to know the miles per minute to miles per hour conversion, I can freely call the static method like this:
Car.milesPerMinute(1);
This will work anywhere, regardless of the number of instances I created, as long as the class is properly imported. The reason is that when you use the static keyword, the method lives on the class itself and not an instance of that class.
This seems like a crappy example, but hope it helps!

Michael North
2,561 PointsThank you very much for these examples. This helps a ton! Also thank you for going through all this trouble to help me out.