Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial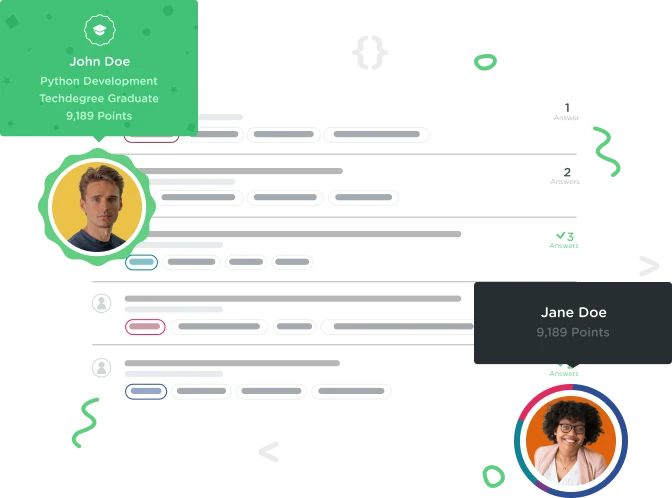

Yan Kozlovskiy
30,427 PointsI don't understand the JS Array Iteration filter video
const names = ['Selma', 'Ted', 'Mike', 'Sam', 'Sharon', 'Marvin'];
const startsWithS = name => name.charAt(0) === 'S'; // I DON'T GET THIS
const sNames = names.filter(startsWithS);
console.log(sNames);
What I don't understand is how JS knows what I am talking about on line 3. I haven't explicitly declared a parameter when I am invoking that arrow function. Name is a parameter and how does JS know that I am talking about without explicitly passing the name i.e. ```sNames = names.filter(name => startsWithS(name));
WHY DO WE DO THIS
const startsWithS = name => name.charAt(0);
INSTEAD OF THIS
sNames = names.filter(name => startsWithS(name));
1 Answer
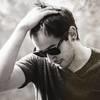
Leonardo Diaz
17,591 PointsDear Yan,
On the second line, "startsWithS" constant was declared a function. This function recieved one parameter called "name", anytime you use this function, the only parameter it may recieve will be called "name" inside this function.
Next, there is the " name.charAt(0) === 'S'; ", this is the only sentence that the function will excecute, in arrow functions this is also a return sentence (When it's only one). This returns a boolean, becouse the sentence is a comparison with 'S'.
And finally, the constant sNames will hold the result of the filter function over the names array. This filter function uses a callback function (you can see that startWithS was used). Filter will iterate the array "names" and give every element of the array as a parameter to the function "startsWithS", whenever the result of the callback function is true, the filter function will store that element of the array on the new const called sNames.
And Also, why don't you declare it the other way. It's just how callback functions work, the parameter goes implicit on the function calling the callback, in this case filter, you don't need to declare that parameter.
When you send just startsWithS you are giving the function as a parameter to filter, and that is what filter asks, for a function. When you say "name => startsWithS(name)", you are overdeclaring the function, or making something like a closure. similar to:
const callback =
function (name) {
var startsWithS = function (name) {
return name.charAt(0) === 'S';
}
return startsWithS;
};
const sNames = names.filter(callback);
When you should be doing something like this:
const callback =
function (name) {
return name.charAt(0) === 'S';
};
const sNames = names.filter(callback);
Remember that arrow functions and anonymous functions asigned to variables work slightly different, but in this case is the same.
If you have more doubts, just ask.