Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial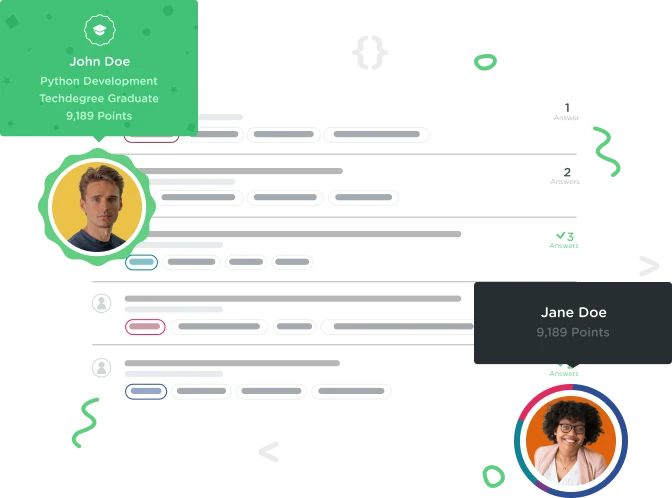

jayda hendrickson
3,413 PointsI don't understand the purpose of super() at all and what it does
I'm completely just confused on the super() function, but none of these explanations are helping, what exactly does super do? And how does the parent class even impact the sneaky attribute??
2 Answers
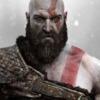
boi
14,242 PointsSince you're a beginner, I'll try to make you understand what you need to understand about super()
, in very simple terms.
consider this code;
import random
class Character:
def __init__(self, name, **kwargs):
self.name = name
for key, value in kwargs.items():
setattr(self, key, value)
Do you see the self.name
attribute in the Character
class? Good, now suppose you want to create another class named Thief
and set an __init__
function with extra attributes also, inheriting everything from the Character
class, so you would probably do something like this 👇
import random
class Character:
def __init__(self, name, **kwargs):
self.name = name
for key, value in kwargs.items():
setattr(self, key, value)
class Thief(Character):
def __init__(self, stealth, endurance, sneaky):
self.stealth = stealth
self.endurance = endurance
self.sneaky = sneaky
Good, now run the program, but wait, there are two __init__
methods, one from the Character
class and other from the Thief
class, since only one __init__
method can run at a time, is there a way to run both of the __init__
methods together? like combined? YES, this is where super()
comes into picture.
To run both of the __init__
methods you can use super()
like this;
import random
class Character:
def __init__(self, name, **kwargs):
self.name = name
for key, value in kwargs.items():
setattr(self, key, value)
class Thief(Character):
def __init__(self, name, stealth, endurance, sneaky, **kwargs):👈#Must include parameters from the inherited class
#in this case those parameters are "name" and "**kwargs"
super().__init__(name, **kwargs) 👈#Used super() here to combine the "Character class __init__" method with
#the "Thief class __init__" method
self.stealth = stealth
self.endurance = endurance
self.sneaky = sneaky
#Notice you don't need to set attributes of the "Character" class here, because you don't need to set it, the super()
#handles that as well
Go into the REPL
>>> from characters import Thief
>>> jayda = Thief("Jayda hendrickson", "42%", "50%", "True")
>>> jayda.name
"Jayda hendrickson"
>>> jayda.stealth
"42%"
>>> jayda.endurance
"50%"
>>> jayda.sneaky
"True"
As you progress further into the course you will understand much more about the usage of super()
, for now try to understand why did Kenneth use it in this video. I recommend you play around with super()
a bit before going further into the course.
Note :This explanation is to give you somewhat idea of the use of super()
, in reality super()
is used when you want to override any method and want to access the version in the parent class.
You should also check out pro Josh Keenan's and the veteran Steven Parker's explanations.

caitlin oloughlin
3,099 PointsThat was incredibly helpful. Thank you!

jayda hendrickson
3,413 PointsThat really helped thank you!
Michael Cook
Full Stack JavaScript Techdegree Graduate 28,975 PointsMichael Cook
Full Stack JavaScript Techdegree Graduate 28,975 PointsDon't psych yourself out of this.
super()
just calls the constructor function on the parent class and gives the inheriting class access to all the properties and methods available on the parent class. That's about it, or that's all you need to know to get going with it at least.