Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial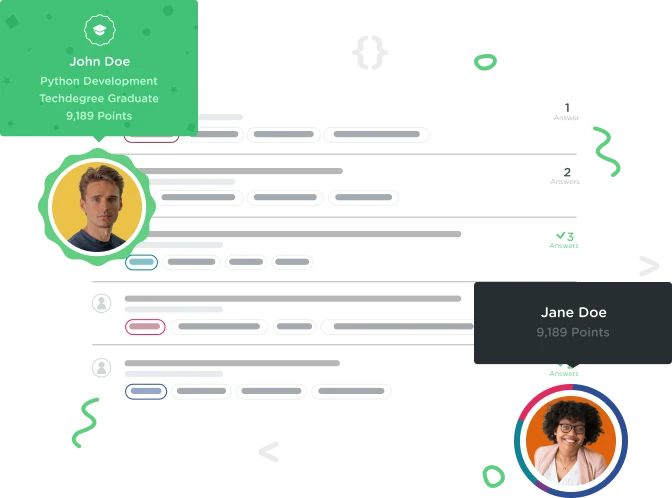
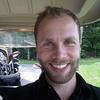
Morten Henriksen
5,443 PointsI don't understand the setup of these last coding questions. The videos seems a bit far from the coding tests.
I feel like there were a lot going on the last two videos. I might just not be doing these consistently enough together, the lessons that is.
I should probably just start over with the entire course, though it just bums me out that I can get this stuck after 4 days away from it.
The question asks to make a method that calls another method and then "normalize" it so it now returns something new. But it doesn't feel the last two videos said anything about changing the returned valued. But instead about making sure BUGs were catched and addressed so wrong input wouldn't put a valid.
I am then asks to change the output, and not catch a BUG.
The teacher also moved coding around, said it was better and it mostly did the same. Though it only confused me even more. How does he know it better? It what way does it affect the program where the lines are. How can I tell when something is not in "the right place".
I definitely need to retake the hole course. It just feels like the learning curve clines so fast. Do I have to read and be comfortable with everything that is in the teachers note? If so, why not just make it part of the course? If I'm expected to either read or research something, why not point it out and say you need to know/learn this, now!, before you continue.
I'm sorry if this has come off harsh, was not my intention. But I felt it would be best to leave it as be.
public class Order {
private String itemName;
private int priceInCents;
private String discountCode;
private String normalizeDiscountCode(String applyDiscountCode) {
discountCode = String.toUpperCase(discountCode);
return this.discountCode;
}
public Order(String itemName, int priceInCents) {
this.itemName = itemName;
this.priceInCents = priceInCents;
}
public String getItemName() {
return itemName;
}
public int getPriceInCents() {
return priceInCents;
}
public String getDiscountCode() {
return discountCode;
}
public void applyDiscountCode(String discountCode) {
this.discountCode = normalizeDiscountCode(discountCode);
}
}
public class Example {
public static void main(String[] args) {
// This is here just for example use cases.
Order order = new Order(
"Yoda PEZ Dispenser",
600);
// These are valid. They are letters and the $ character only
order.applyDiscountCode("abc");
order.getDiscountCode(); // ABC
order.applyDiscountCode("$ale");
order.getDiscountCode(); // $ALE
try {
// This will throw an exception because it contains numbers
order.applyDiscountCode("ABC123");
} catch (IllegalArgumentException iae) {
System.out.println(iae.getMessage()); // Prints "Invalid discount code"
}
try {
// This will throw as well, because it contains a symbol.
order.applyDiscountCode("w@w");
}catch (IllegalArgumentException iae) {
System.out.println(iae.getMessage()); // Prints "Invalid discount code"
}
}
}
1 Answer

Benjamin Deollos
2,049 PointsMorten, I would have to agree with you when you state that he jumps around a lot in the videos. Unfortunately that is part of coding. Regarding your issues with the code and completing the challenge, here are some hints that I can give you hopefully they work for you.
public class Order {
private String itemName;
private int priceInCents;
private String discountCode;
//in the argument (String applyDiscountCode) remember that it is returning nothing hense the void.
private String normalizeDiscountCode(String applyDiscountCode) {// You are wanting to change your argument
discountCode.
//Here you are going to want to perform a for loop checking the characters in the discountCode.
for (char character : discountCode.toCharArray()) {
//then an if statement checking the letters and the one char '$'
if (!(Character.isLetter(character)) && (character != '$')) {
//Here you throw your new illegal argument exception if it is not a letter and does not contain a '$'
throw new IllegalArgumentException("Invalid discount code");
}
}
discountCode = String.toUpperCase(discountCode); //this line is not needed due to you already assigning
//this.discountCode = normalizeDiscountCode(discountCode) in
//the applyDiscountCode();
return this.discountCode; //Here you can just return the discountCode and convert it to upper case with the
//.toUpperCase()
}
public Order(String itemName, int priceInCents) {
this.itemName = itemName;
this.priceInCents = priceInCents;
}
public String getItemName() {
return itemName;
}
public int getPriceInCents() {
return priceInCents;
}
public String getDiscountCode() {
return discountCode;
}
public void applyDiscountCode(String discountCode) {
this.discountCode = normalizeDiscountCode(discountCode); //assigning this instance of discountCode to
//normalizeDiscountCode();
}
}