Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial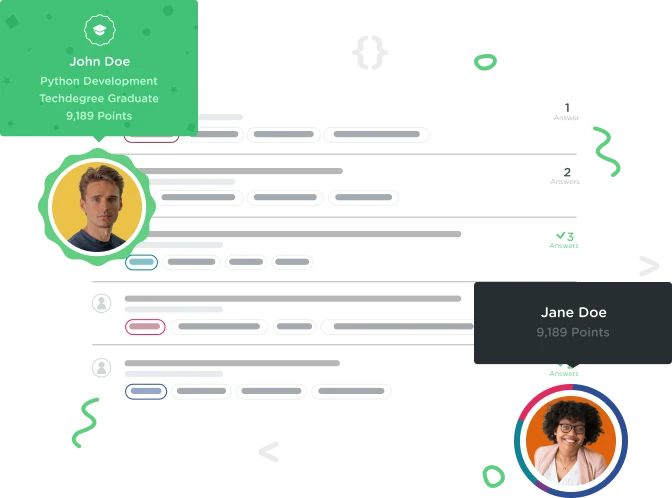

Joseph Chauvin
Full Stack JavaScript Techdegree Student 12,029 PointsI don't understand this question at all and this whole course has just been weird to me
I'm not sure why this code doesn't work for the challenge
const customers = [
{
name: "Tyrone",
personal: {
age: 33,
hobbies: ["Bicycling", "Camping"]
}
},
{
name: "Elizabeth",
personal: {
age: 25,
hobbies: ["Guitar", "Reading", "Gardening"]
}
},
{
name: "Penny",
personal: {
age: 36,
hobbies: ["Comics", "Chess", "Legos"]
}
}
];
let hobbies;
// hobbies should be: ["Bicycling", "Camping", "Guitar", "Reading", "Gardening", "Comics", "Chess", "Legos"]
// Write your code below
hobbies = customers
.map( person => person.personal.map( person => person.hobbies ))
.reduce( (arr, person) => [ ...arr, ...person], []);
2 Answers

Seth Kroger
56,413 PointsThe trouble you're having is that person.personal
is an object, not an array so there is no map function. Since there is only one hobbies
entry in personal
the map part can just be:
.map( person => person.personal.hobbies )

mc24
Courses Plus Student 3,041 Pointsconst customers = [
{
name: "Tyrone",
personal: {
age: 33,
hobbies: ["Bicycling", "Camping"]
}
},
{
name: "Elizabeth",
personal: {
age: 25,
hobbies: ["Guitar", "Reading", "Gardening"]
}
},
{
name: "Penny",
personal: {
age: 36,
hobbies: ["Comics", "Chess", "Legos"]
}
}
];
let hobbies;
// hobbies should be: ["Bicycling", "Camping", "Guitar", "Reading", "Gardening", "Comics", "Chess", "Legos"]
// Write your code below
hobbies = customers[0].personal.hobbies
hobbies += customers[1].personal.hobbies
hobbies += customers[2].personal.hobbies
console.log(Array(hobbies));
I am still at the Javascript basics, just wanted to solve that for me. I dont know if this method helps you, but Hobbies is now that what it should be =).

Joseph Chauvin
Full Stack JavaScript Techdegree Student 12,029 PointsI have to use the reduce method :/