Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial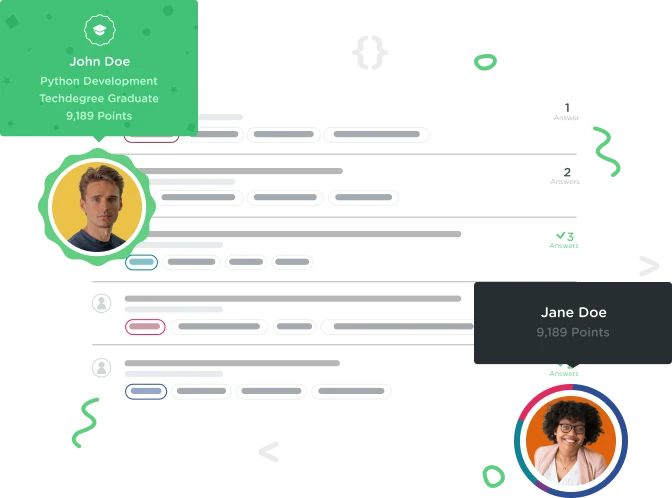

Marius Sandli
1,097 PointsI dont understand this task, help
I need help asap
public class ConferenceRegistrationAssistant {
public int getLineFor(String lastName) {
/* If the last name is between A thru M send them to line 1
Otherwise send them to line 2 */
boolean lineOne = lastName.charAt(0) && lastName. ;
if(lineOne.contains('A' < 'M'){
int line = 1;
}else{
int line = 2;
}
int line = 0;
return line;
}
}
2 Answers
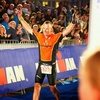
Steve Hunter
57,712 PointsHi Marius,
You want to use the parameter lastName
that is passed in. You're concerned about it's first letter so you can use .charAt(0)
to isolate that.
Once you have the first letter, you need to test if it is 'M' or less. If it is, set the line variable to be 1. Else, set it to 2.
Then return line
. That could look something like:
public class ConferenceRegistrationAssistant {
public int getLineFor(String lastName) {
/* If the last name is between A thru M send them to line 1
Otherwise send them to line 2 */
int line = 0;
if(lastName.charAt(0) <= 'M'){
line = 1;
} else {
line = 2;
}
return line;
}
}
I hope that helps.
Steve.

Rachelle Wood
15,362 PointsYou are almost there! You are just overcomplicating things a little. You don't need to declare a Boolean at all in order to do this, you just need a simple if statement that compares the first character of your lastName string to the values A and M. One big important thing to know though is that return has to be the last line in the function and your variable value has to be set before you do anything.
public class ConferenceRegistrationAssistant {
public int getLineFor(String lastName) {
/* If the last name is between A thru M send them to line 1
Otherwise send them to line 2 */
int line = 0;
if (lastName.charAt(0) >= 'A' && lastName.charAt(0) <= 'M') {
line = 1;
} else {
line = 2;
}
return line;
}
}

Rachelle Wood
15,362 PointsDrop the first condition I wrote, just needs to be less than or equal to M.

Marius Sandli
1,097 PointsThanks alot :)