Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial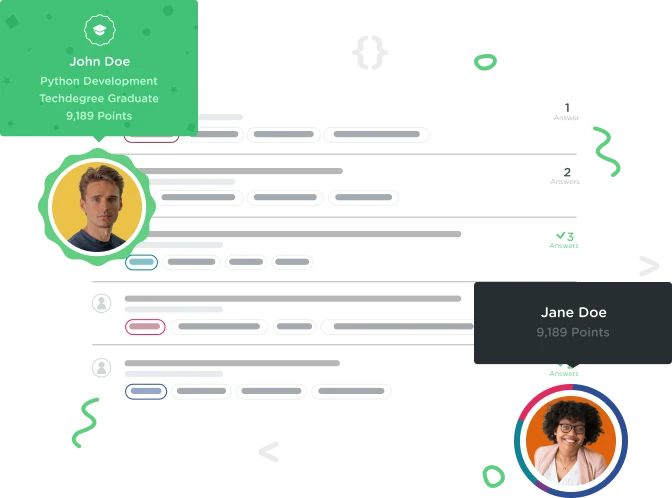

Brandon Allen
2,383 PointsI Don't Understand this While Loop
The challenge tells me that my syntax for this while loop is correct, but I know its not. Could someone explain this a little further.?
var secret;
while ( secret !== "sesame" ) {
secret = prompt("What is the secret password?");
}
document.write("You know the secret password. Welcome.");
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title>JavaScript Loops</title>
</head>
<body>
<script src="app.js"></script>
</body>
</html>
2 Answers
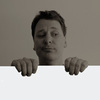
Sean T. Unwin
28,690 PointsThis is correct.
If you open your browser's dev tools and type the following into the Console:
var secret;
while ( secret !== "sesame" ) {
secret = prompt("What is the secret password?");
console.log('secret', secret);
}
You will see that a prompt will keep popping until you enter in 'sesame'. The console.log()
statement will display in the console whatever you entered into the prompt.
The while
loop will do whatever it's told until the condition is met. This can be helpful, yet in certain instances dangerous, because, generally, unlike a for
loop, if the while
condition is not worded, or structured, properly (or the body of the loop does not have sufficient error trappings) it could cause an indefinite loop. For example, in this scenario if the user never inputs 'sesame' the popup will continue to display after each entry.

Brandon Allen
2,383 PointsI think I figured it out, but can you tell me if this is correct:
var secret;
while ( secret !== "sesame" ) {
secret = prompt("What is the secret password?");
}
document.write("You know the secret password. Welcome.");
Ira Bradley
12,976 PointsIra Bradley
12,976 PointsYour code is correct.
The statement that the while loop evaluates is (secret !== "sesame"). In plain english this could be written as "While the variable 'secret' does not equal the string 'sesame' do the following:
ask (and store the answer in the variable 'prompt') - "What is the secret password?".
You do not exit the while loop until what is typed in is "sesame" when the user is prompted. When the user enters the correct password, "You know the secret password. Welcome." is printed to the screen. Let me know if this doesn't make sense.