Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial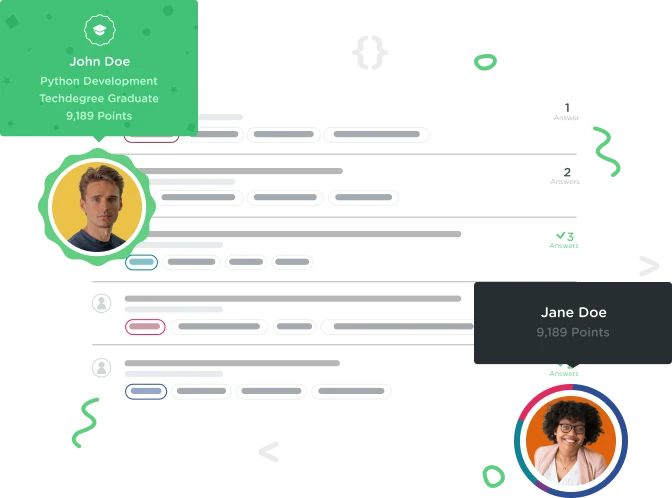

Kshatriiya .
1,464 PointsI don't understand Treet originalTreets = treets[0]
Hello what is the purpose of declaring arrays with length of 0?
Here's another one:
Treet[] treets = new Treet[0];
As I understand it, Arrays are fixed length, if you declare a fixed length array you won't be able to put anything into it?
2 Answers
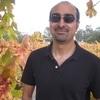
Kourosh Raeen
23,733 PointsThe purpose of that line is to create an empty array that can hold the return value of the readObject() method, which is an array of Treets. It is true that the array is fixed length but you are just changing the reference here. However if you tried something like this:
Date date = Calendar.getInstance().getTime();
treets[0] = new Treet("The author", "The description", date);
then you get an ArrayIndexOutOfBoundsException exception when you run the program. Take a look at this code as simple example you can try:
public class ForumQuestion {
public static void main(String[] args) {
String[] emptyArray = new String[0];
String[] otherArray = {"first", "second", "third"};
//emptyArray[0] = "item1";
emptyArray = otherArray;
System.out.println("Items in otherArray:");
for (String item : otherArray) {
System.out.println(item);
}
System.out.println("\nItems in emptyArray:");
for (String item : emptyArray) {
System.out.println(item);
}
}
}
Here emptyArray is just an empty array and otherArray is an array of size 3, containing three strings. I can assign otherArray to emptyArray, which means that both object references will point to the same Array object, as you can see from the printout also.
Now, if you uncomment the line
emptyArray[0] = "item1";
and recompile and run, then you get ArrayIndexOutOfBoundsException exception.
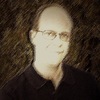
Jason Anders
Treehouse Moderator 145,860 PointsHey there,
So, with the first line of code you gave, Craig actually isn't declaring an array (or length of).
Treet originalTreet = treets[0]
Here, the 0
is being used as an index number and not the length of the List. Here Craig is taking the value at the Zero index in the treets ArrrayList to the originalTreet
variable. So, the first Treet written.
With the second example you gave, if I remember correctly Treet[]
is an ArrayList
, which is a "Resizable-array implementation of the List interface".
Treet[] treets = new Treet[0];
So, here Craig is implementing an instance of the Treet List to be used to store the actual Treets. He starts of with just initializing an empty List, but can and will soon add to it.
I'm still relatively new to Java, so I hope this makes sense. Keep Coding!
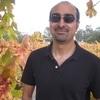
Kourosh Raeen
23,733 PointsHi Jason - Actually, Treet[] is not an ArrayList. It's just a regular array of objects, in this case objects of type Treet. You can see my explanation above as why the following line works:
treets = (Treet[]) ois.readObject();
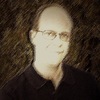
Jason Anders
Treehouse Moderator 145,860 PointsThanks Kourosh Raeen
The video referenced is titled "Array Lists", so I just went with that. I had just recently watched that video, but could not recall when Craig created the Treet
object in the first place. I remember him importing List
and ArrayList
, I guess I will go back and re-watch the last couple of videos. :)
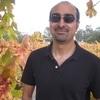
Kourosh Raeen
23,733 PointsI think a better place for this question would be under the Serialization video since that's where Craig writes the code in question.
Kshatriiya .
1,464 PointsKshatriiya .
1,464 PointsHello, thank you for your help, I'm still really confused as to what you mean by reference.
I ran your code and have found that emptyArray is able to get the items in otherArray despite being given a length of 0.
I'm told that Arrays are not flexible but then how come emptyArray can expand itself to take 3 items from otherArray?
Wouldn't it make sense to just write:
String[] emptyArray = new String[];
instead.
Again thank you for your help.
Edit: I'm coming to the conclusion that emptyArray hasn't expanded, instead by equating it to the otherArray you've pointed its reference object to otherArray's reference object instead?
In that case, am I correct in assuming that
new String[0]
is sort of like a place holder object?
Kourosh Raeen
23,733 PointsKourosh Raeen
23,733 PointsYou are correct that emptyArray hasn't expanded. You are just getting it to point to a different place in the memory, the same place in memory that otherArray is pointing to.
Regarding your comment about declaring emptyArray like this:
String[] emptyArray = new String[];
That's not going to work. You will get an array dimension missing error at compile time. If you are using the new keyword to create an array you need to give it a dimension, hence the zero.