Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial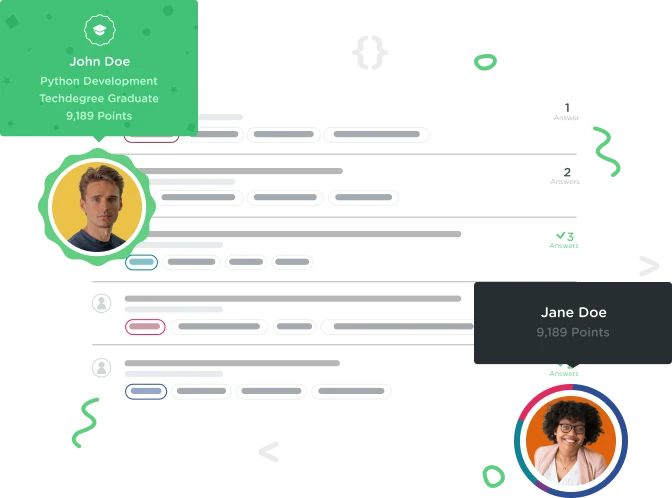

Florenci Viela
2,482 PointsI don't understand what do they mean by "Pass it a 1" in the second question of the code challenge.
It might be because English is not my first language, but I can't figure out what do they mean by that, so I can't complete the task. I've been stuck for a while already.
Thank you!
class GoKart {
public static final int MAX_BARS = 8;
private String color;
private int barCount;
private int lapsDriven;
public GoKart(String color) {
this.color = color;
}
public String getColor() {
return color;
}
public void charge() {
barCount = MAX_BARS;
}
public boolean isBatteryEmpty() {
return barCount == 0;
}
public boolean isFullyCharged() {
return MAX_BARS == barCount;
}
public void drive(int laps) {
lapsDriven+= laps;
barCount-= laps;
}
public void drive(){
drive = 1;
}
}
3 Answers
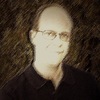
Jason Anders
Treehouse Moderator 145,860 PointsHi Florenci,
"Pass it a 1" means to provide the function's parameter(s) with a value (or arguments). In this case, you have created the method:
public void drive(int laps) {
lapsDriven+= laps;
barCount-= laps;
}
This method "takes" one parameter (an Integer named "laps"). In short, when you call the method, you "pass in" an argument (the value), so the method can do what it's programmed to do. Here, the integer you pass in will be used to complete the calculations on lapsDriven
and barCount
. So, whatever number you pass into the method when you call it, will increase the lapsDriven
and decrease the barCount
by that number.
Remember to call a method is simply by typing the name of the method followed by opening and closing parenthesis with the arguments inside.
Now Task two asks you to create a another method that takes no parameters (and you did this correctly), but the second part wants you to call the first drive
method, passing in a 1. Just a quick note, although these methods are named the same, they are different because they have different signatures (the parameters required).
So, as stated above: to call a method is just typing the name and () with the arguments passed in between.
public void drive(){
drive(1);
}
I hope this helps. The more you work with overloading methods, the more it will makes greater sense.
Keep coding! :)
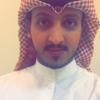
Fahad Mutair
10,359 Pointshi Florenci Viela ,
Create a new method named drive that accepts no arguments. It should call the newer drive method passing in a 1 for the default.
Create a new method named drive that accepts no arguments means another method with same name drive (Overloading)
public void drive()
It should call the newer drive method passing in a 1 for the default. so in the body of the new method we have to call the other method that accept laps parameter
drive(1);
so the final code is
public void drive() {
drive(1);
}

Florenci Viela
2,482 PointsThank you so much guys! This community is amazing, I posted the question 25 minutes ago and already have the answer.
Casey Bryant
619 PointsCasey Bryant
619 PointsEnglish is my first language and I didn't understand that part either ;) Thanks for helping!