Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial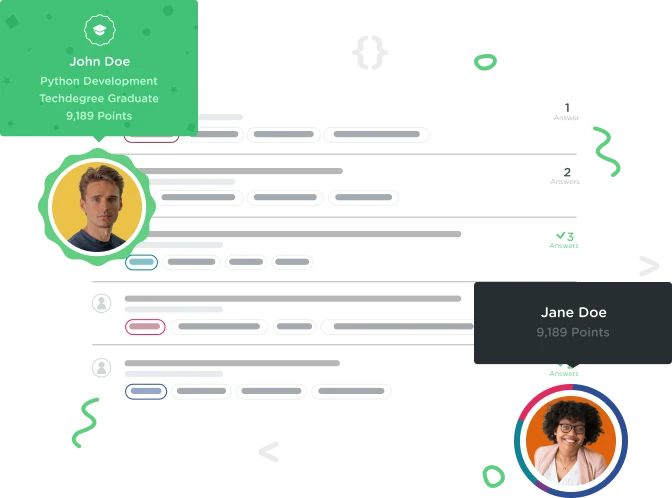

Sam Grumley
1,765 PointsI don't understand what goes between "%" + ?? + "%"
I don't understand what goes between the placeholders i have tried every variable from both files including objects.
var utilities = require("./utilities");
var mailValues = {};
mailValues.first_name = "Janet";
var emailTemplate = "Hi %first_name%! Thanks for completing this code challenge :)";
var mergedContent = utilities.merge(emailTemplate, mailValues);
//mergedContent === "Hi Janet! Thanks for completing this code challenge :)";
function merge(content, values) {
content = content.replace( "%" + values.emailTemplate + "%" ,values.mailValue);
return content;
}
module.exports.merge = merge;
3 Answers

Umesh Ravji
42,386 PointsHi Sam, the values
given to the merge
method are an object
which means that you have to iterate over all of it's key/value pairs to replace all the placeholders
with thier correct values.
What you want to end up with inside your merge
function is essentially content.replace('%first_name%', 'Janet')
. This can be achieved by surrounding the key
with percentages
on both sides (placeholder), and replacing that placeholder
with its value from the object.
function merge(content, values) {
for (var key in values) {
content = content.replace('%' + key + '%', values[key]);
}
return content;
}

andren
28,558 PointsThe name of the placeholder is what goes in between the %%, in the example above that would be first_name
but your method should be able to handle a string with arbitrary placeholder names.
To do this you have to loop over the values
object you are passed in to get access to the names of its properties (since that will correspond to the names of the placeholders), then use that name to pull the desired value out of the object. Like this:
function merge(content, values) {
for(var key in values) {
content = content.replace( "%" + key + "%" , values[key]);
}
return content;
}
module.exports.merge = merge;
With that code you can pass a string with any placeholder you want, for example:
"Hi %firstName% %lastName% congratulations for winning our lottery prize on %date%."
And as long as the object passed in contained values for those placeholders your function would replace them without any issue.

Sam Grumley
1,765 PointsAhh yes, thanks heaps. The concept makes heaps more sense!
Emmanuel Molina
9,268 PointsEmmanuel Molina
9,268 PointsYeah, but why create a loop if there is only one key to replace ?
Why
function merge(content, values) { content = content.replace("%first_name%", values.first_name); return content; }
doesn't work ?andren
28,558 Pointsandren
28,558 PointsThat would not work because the challenge is not to build a function that only accepts one specific placeholder, the challenge is to build a function where:
The code in the
index.js
file is just there to show how the input to your function will be formatted and how the returned content should be formatted. But it is not the only input your function will be expected to handle, any placeholder and any number of placeholders should work.So not only should this code work:
utilities.merge("Hello there %first_name%", {first_name: "Janet"});
But something like this code should work as well:
utilities.merge("Hi %first_name% %last_name% congratulations for winning our lottery prize on %date%.", {first_name: "Janet", last_name: "Smith", date: "10.08.17"});
And anything else along those lines.