Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial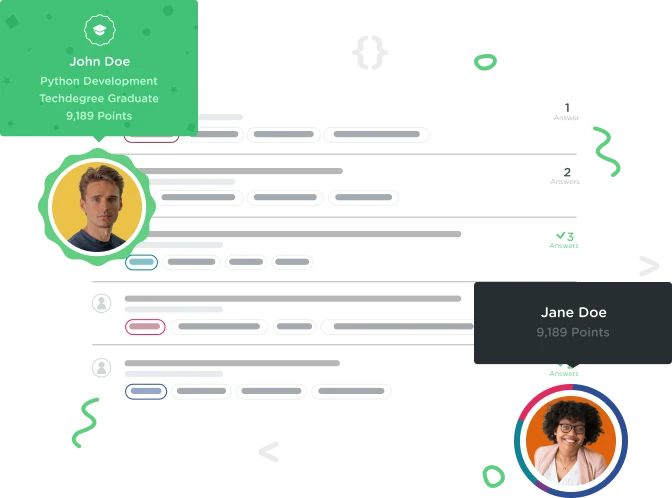

Sandu Gabriel
2,563 PointsI don't understand what I have to do to get this problem solved
I do not understand the question really
import re
string = '''Love, Kenneth: 20
Chalkley, Andrew: 25
McFarland, Dave: 10
Kesten, Joy: 22
Stewart Pinchback, Pinckney Benton: 18'''
players = re.search(r'''
(?P<last_name>[\w]+\s?[\w]+?),
\s(?P<first_name>[\w]+\s?[\w]+?):
\s(?P<score>\d{2})
''', string, re.MULTILINE | re.VERBOSE)
class Player:
def __init__(self, text, matches):
for match in matches.finditer(text):
self.last_name = match.group('last_name')
self.first_name = match.group('first_name')
self.score = match.group('score')
2 Answers

Øyvind Andreassen
16,840 PointsHey Sandu Gabriel,
I think you're overthinking this. Re-read what the question actually asks.
Create a variable named players that is an re.search() or re.match() to capture three groups: last_name, first_name, and score. It should include re.MULTILINE.
There is no mention of creating an object for each of the players, just to catch them in one variable.
You're regex is also a bit of, I always use regex101.com when I write regex since they break it down for you.
A correct pattern for this challenge is:
^(?P<last_name>\w*\s?\w*)\,\s(?P<first_name>\w*\s?\w*)\:\s(?P<score>\d*)$
Let's examine what this pattern looks for: Last Name: At the start of the line look for a words of any length but with an optional whitespace and a following word of any length. The look for a comma and whitespace. First Name: Same pattern as Last Name. Then look for colons and a whitespace. Score should be a number of any length. Then we are at the end of the line.
So the solution can look like this, and will pass the challenge.
players = re.search(r'''
^(?P<last_name>\w*\s?\w*)\,\s(?P<first_name>\w*\s?\w*)\:\s(?P<score>\d*)$
''', string, re.X | re.M)
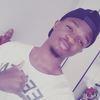
Sean Coutinho
7,788 PointsHey nice code andreeason. Could you also help me with this? Why does my code give an 'players doesn't seem to be a regex object' errror? Thanks in advance
import re
string = '''Love, Kenneth: 20
Chalkley, Andrew: 25
McFarland, Dave: 10
Kesten, Joy: 22
Stewart Pinchback, Pinckney Benton: 18'''
players = re.match(r'''
^(?P<last_name>-?\w*\s?\w*)
\,\s
(?P<first_name>-\w*\s\w*)
\:\s
(?P<score>\d{2})$
''', string, re.X|re.M)
Perfect Tinotenda Mashingaidze
5,070 PointsPerfect Tinotenda Mashingaidze
5,070 Pointsthanks happy coding.....