Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial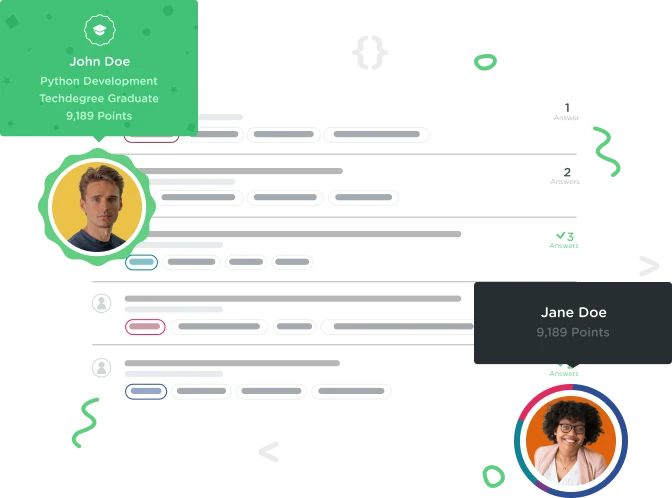

Andrew Winkler
37,739 PointsI don't understand what IEnumerable<int> implies. LINQ help please.
Well, here is my code. The lesson is not very similar at all to this exercise other than the query itself.
using System.Collections.Generic;
using System.Linq;
namespace Treehouse.CodeChallenges
{
public class NumberAnalysis
{
private List<int> _numbers;
public NumberAnalysis()
{
_numbers = new List<int> { 2, 4, 6, 8, 10 };
}
public NumbersGreaterThanFive()
{
//declare the new IEnumerable<int>
IEnumberable<int> listGreaterThanFive = new IEnumerable<int>();
//assign it to the query
listGreaterThanFive = for number in _numbers where number > 5 select number;
// return it
return listGreaterThanFive;
}
}
}
2 Answers
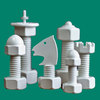
Steven Parker
231,275 PointsIEnumerable<int> is just a collection type, a bit like a List, but more primitive (List inherits from it).
I see a few issues in the code:
- You forgot to declare the return type ("IEnumerable<int>") of the method
- You spelled "IEnumberable" (instead of "IEnumerable") in one place
- You don't need to call an explicit constructor for listGreaterThanFive

Janice Childers
18,958 PointsCan anyone help with this, please? I am also stuck on this as it is completely different than the lesson except for the query itself.