Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial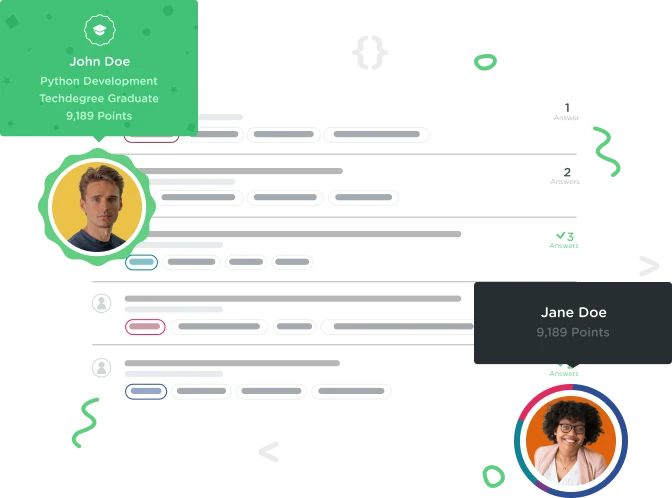

Julianna Kahn
20,702 PointsI don't understand what is meant by assigning an "anonymous" function.
Maybe I am a little confused on my terminology? The function is the same as the event handler?
var xhr = new XMLHttpRequest();
xhr.onReadyStateChange = function() {
if(xhr.ReadyState === 4);
alert(xhr.statusText);
}
xhr.open('GET', 'sidebar.html');
xhr.send();
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title>AJAX with JavaScript</title>
<script src="app.js"></script>
</head>
<body>
<div id="main">
<h1>AJAX!</h1>
</div>
<div id="sidebar"></div>
</body>
</html>
4 Answers
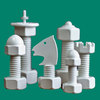
Steven Parker
231,268 PointsThe "anonymous" part just means that the function is created in an expression where it is not given a name. It doesn't need one since the code has another way to reference it.
The instructions say to "add an onreadystatechange event handler.", You did add an event handler, but you assigned it to a property named "onReadyStateChange" (with mixed case) instead of "onreadystatechange" (all lower case).
Also, while it won't affect passing, the instructions also said to add the function "but don't put any code inside it."
And this will also be ignored by this challenge, but just FYI: for an "if" to work, you can't put a semicolon after the conditional clause.

Julianna Kahn
20,702 PointsI thought that would mean function().
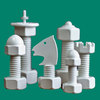
Steven Parker
231,268 PointsThat's exactly what you have on the second line:
xhr.onReadyStateChange = function() { // <- anonymous function

Julianna Kahn
20,702 PointsI thought before that it might be but I still get an error:
Bummer: There is no event handler for the onreadystatechange event. You can set the event handler by assigning an anonymous function to xhr.onreadystatechange.
var xhr = new XMLHttpRequest(); xhr.onReadyStateChange = function() { } xhr.open('GET', 'sidebar.html'); xhr.send();
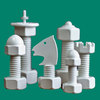
Steven Parker
231,268 PointsOh, I thought the question was just about anonymous functions in general. I expanded my original answer.
Does that help?

Julianna Kahn
20,702 PointsYes, your explanation helps a lot. The if statement was just something I was trying to test if that was why I was getting the error, but I guess not. And I understand that the semicolon didn't help.