Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial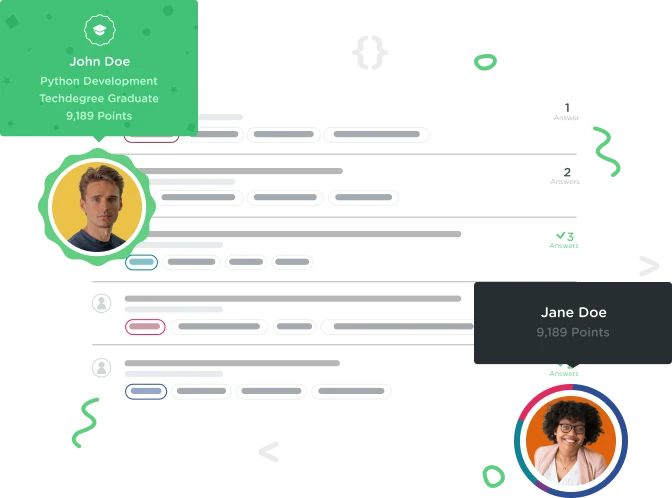
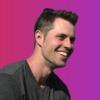
Michael Sothan
8,048 PointsI don't understand what is the question I'm trying to solve for this problem
My understanding is "Allow the user to designate the amount of additional laps the GoKart will drive when calling the drive method." However, the hints are talking about decrementing the barCount using -=. I don't see how that's related to the question at all. Please elaborate on what we are trying to achieve. Thanks
class GoKart {
public static final int MAX_BARS = 8;
private String color;
private int barCount;
private int lapsDriven;
private final int TOTAL_LAPS;
public GoKart(String color) {
this.color = color;
}
public String getColor() {
return color;
}
public void charge() {
barCount = MAX_BARS;
}
public boolean isBatteryEmpty() {
return barCount == 0;
}
public boolean isFullyCharged() {
return MAX_BARS == barCount;
}
public void drive() {
lapsDriven++;
barCount--;
}
public void drive() {
drive(TOTAL_LAPS);
}
public void drive(int laps2Go) {
lapsDriven += laps2GO;
}
}
1 Answer

Yanuar Prakoso
15,196 PointsHi Michael
This is the goal of the task 1 of 2: Some users of our GoKart class wrote and asked that our drive method accept a parameter to specify how many laps to go, instead of just one.
Yes it means we want the users to be able to designate their requested laps. Therefore they do not have to call drive() method each time they want to go for ONE lap. As you know that for each lap two variables will change right? The number of bars in the battery (barCount) and the number of laps driven (lapsDriven). In previous drive() method since it only runs for one lap meaning the barCount will only decremented one time (barCount -- ) and the lapsDriven will be only incremented one time (lapsDriven ++).
However in this challenge the users get to decide how many laps they want to Drive. Meaning the barCount will be reduced more than one lap if the users want more than one lap right? So naturally does the number of laps driven. Lucky for us the number of bars in the battery (barCount) is decremented exactly One bar for each lap. Thus the number of barCount decremented is exactly how much laps is requested (barCount -= lapsRequested). So does the number od lapsDriven incremented to how much laps requested (lapsDriven += lapsRequested). This how I wrote my code:
public void drive(int lapsRequest) {
lapsDriven += lapsRequest;
barCount -= lapsRequest;
}
PS: for the task 1 of 2 you just need to modify the public void drive() method. You do not need to add another drive() method yet since it is not necessary yet at this moment. It will in the task 2 of 2 after this which method overloading is used.
I hope this will help a little.
Michael Sothan
8,048 PointsMichael Sothan
8,048 PointsThanks!
I actually figured it out on my own after I submitted the question (when I finally thought about connecting the barCount to the lapsDriven in the second drive method in a 1:1 relationship) but wouldn't have analyzed in that way if I didn't submit the question in the first place! I was thinking if I was going to modify two variables (barCount and lapsDriven) that I needed to pass two parameters to the drive method inside the body of drive() or make two separate calls to the other drive method. Now I realize I can just pass one parameter with one call and just need to include both conditionals in the second drive method.
Anyway, thanks for the detailed response. I think you telepathically steered me to shore even before you answered!