Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial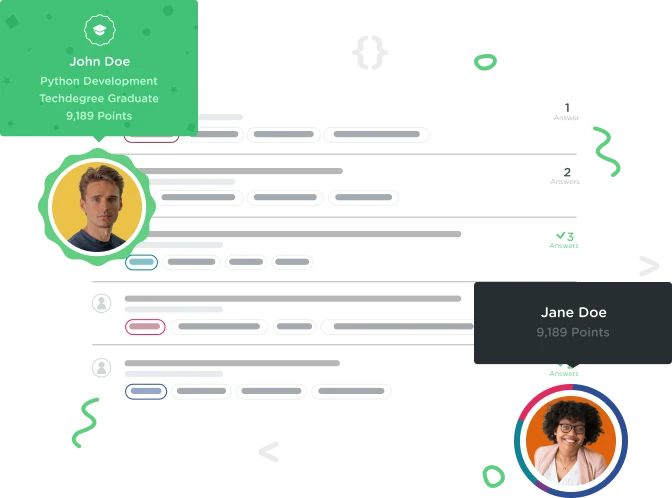

Henry Miller
7,945 PointsI don't understand what it wants me to do?
This is the challenge in finding array items and I don't get it. Please help? See my code below.
class TodoList
attr_reader :name, :todo_items
def initialize(name)
@name = name
@todo_items = []
end
def add_item(name)
todo_items.push(TodoItem.new(name))
end
def find_index(name)
index = 0
found = false
if found
todo_items[index]
return true
else
return nil
end
end
end
2 Answers
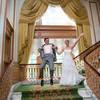
Colin Bell
29,679 Points- You have to loop through each item first and check each item's
name
vs thename
given. - If the names match, set
found
equal to true and then break out of the each loop - no need to keep looking once you've already found it. - If it is not found, add one to the
index
. Then it will start the loop over with the nexttodo_item
until there aren't any items left to check. - If the todo_item was found, return the
index
- If after every
todo_item
intodo_items
has been checked, and found wasn't true then returnnil
def find_index(name)
index = 0
found = false
todo_items.each do |todo_item| #1
if todo_item.name == name #2
found = true #2
end
if found #2
break #2
else
index += 1 #3
end
end
if found #4
return index#4
else
return nil #5
end
end
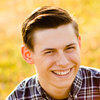
Caleb Kleveter
Treehouse Moderator 37,862 PointsIsn't there a better way to do that? Or is there not?
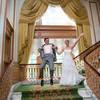
Colin Bell
29,679 PointsI'm sure it can be optimized, but I just pulled the code straight from the video and broke down what each portion was doing.
Something like this might cut down a few lines of code, though I didn't test it:
def find_index(name)
index = 0
found = false
todo_items.each do |todo_item| #1
if todo_item.name == name #2
found = true #2
break
else
index += 1 #3
end
end
if found
return index #4
else
return nil #5
end
end
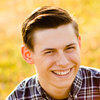
Caleb Kleveter
Treehouse Moderator 37,862 PointsOkay, thanks!
Henry Miller
7,945 PointsHenry Miller
7,945 PointsThanks!