Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial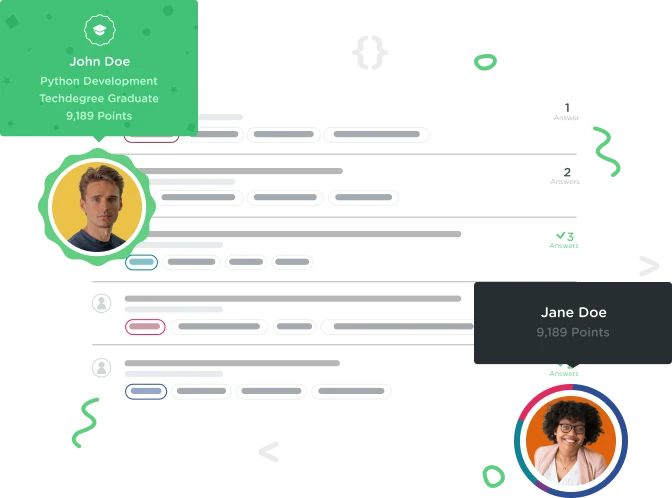
Jeff Ward
8,978 PointsI dont understand what "module.exports.get = get;" does? Can someone please elaborate?
I dont understand what "module.exports.get = get;" does?
Can someone please elaborate on what this does and why I need it?
Why does the code not execute without it?
Thanks!
app.js:
var profile = require("./profile.js");
profile.get("jeffward2");
profile.js:
//Problem: we need a simple way to look at a user's badge count and Javascript points
//Solution: Use node.js to connect to Treehouse's API to get progile information and print out
var http = require("http");
//Print out message
function printMessage(username, badgeCount, points) {
var message = username + " has " + badgeCount + " total badge(s) and " + points + " points in JavaScript.";
console.log(message);
}
//Print out Error messages
function printError(error) {
console.error(error.message + "<---- error has occured!. Oh Nooz!");
}
function get(username) {
//Connect to API URL (https://teamtreehouse.com/username.json)
var request = http.get("http://teamtreehouse.com/" + username + ".json", function (response) {
var body = "";
//Read the data
response.on('data', function (chunk) {
body += chunk;
});
response.on("end", function () {
if (response.statusCode === 200) {
try {
//Parse the data
var profile = JSON.parse(body);
//Print the data out
printMessage("jeffward2", profile.badges.length, profile.points.JavaScript);
} catch (error) {
//Parese Error
printError(error);
}
} else {
printError({
message: "There was an error getting the profile for " + username + ". (" + http.STATUS_CODES[response.statusCode] + ")"});
}
});
});
//Check for connection error
request.on("error", printError);
}
module.exports.get = get;
4 Answers
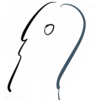
akak
29,445 PointsIn your app.js file you used a function get (profile.get("jeffward2");) written in another file - profile.js. By export, you've allowed function get from profile.js to be used in app.js. Without it, by just requiring file profile.js, you wouldn't be able to call it in app.js.
Jeff Ward
8,978 PointsSo by saying:
module.exports.get = get;
For clarification: I am saying that this function 'get' is available to be used and called on by all other JavaScript files that require profile.js. Otherwise if its not declared with the .exports, it cannot be utilized by other files?

Jarrad O'Brien
8,136 PointsJust in case anyone checks this question out, you can imagine this line:
var profile = require("./profile.js");
As jamming the entire contents of 'profile.js' into the variable profile. So to access anything in the variable profile, you need to do 'profile.x' similar to when you create an object and want to access any properties or variables.
'module.exports.x = x' is a line that just says that we want to make the the right side available for access, and not just hidden inside of 'profile'.
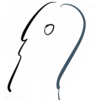
akak
29,445 PointsYes :)

Ognjen Jevremovic
13,028 PointsJust a heads up, I was trying to explain this to myself as well.
How I manged to understand it as I thought of it as being the same concept used in the prototypal inheritance, when working with creator function in JavaScript. Not quite sure if you did that course already here on TreeHouse, but basically you're "prototyping" the method that can be shared across all of the instances of an object, created through that same creator function.
If you went through that course, I think you'll be able to find the same analogy I followed, or it's just how I understood it; judging by the akak's response, it seems like that it's not that far from the truth = ). As in both, you're exporting the method to be used either on another instance of that object, or in this case on a module that you're requiring (with require()
) later on or in other file.
I'm used to learning things this way, as it helps me remember much easier and at the same time I'm relearning the concepts from before, that I perhaps forgot by the time.
Cheers! And happy coding
Update: After watching the video for the second time I've came to one more conclusion on my own, on why the methods on the module must be exported (I am not saying it's 100% correct, but it just helped me understand it better and it will perhaps someone else too!).
When we're programming on the web, more particularly for the web browser (javascript code that will get executed on the client side) we have HTML document in which we're linking to the javascript files (by using script tags), which allows us to use a code from one javascript file, inside of another, ONLY if the javascript file from which we're requiring the code (functions, variables, etc) is linked first. To explain it little further, a simple example we're all familiar with would look like this:
<script src="jQuery.js"></script>
<script src="app.js"></script>
So if we want to use the jQuery methods in our code, we must link to the jQuery library first, before we link to our own app.js file.
Since the Node is a console driven and there's no HTML in which we actually do link the javascript files in following a certain order, we need to explicitly say that we want to use a function (aka method) from one file in our other files, which the module.exports.methodName
will actually allow us to.
Correct me if I'm wrong please, since I don't want to be posting inaccurate data =p. Hope that helps.
Chyno Deluxe
16,936 PointsChyno Deluxe
16,936 Points//Fixed Code Presentation