Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial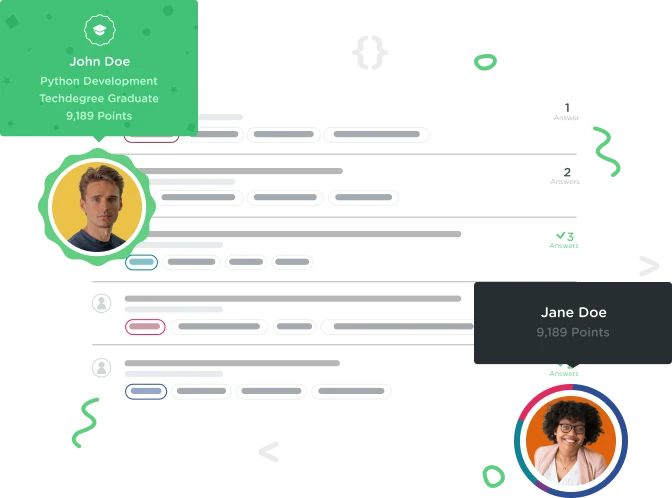

Tristan bradford
1,123 PointsI don't understand what they're looking for
I know my code will work in the way they asked, but I'm not sure how to go about this problem using loops, or I don't know what answer they're looking for.
num_list = list([1, 2, 3])
add_list = sum(num_list)
summarize = "The sum of " + str(num_list) + " is " + str(add_list)
3 Answers

Zachary Hudson
Courses Plus Student 12,154 PointsI'm going to let Kevin break this down if I did it wrong, but this passed for me after retrying.
def add_list(list):
total = 0
for number in list:
total += number
return total
def summarize(list2):
total2 = 0
for number in list2:
total2 += number
list2string = ''.join(str(list2))
return "The sum of {} is {}".format(list2string, total2)
So the real issue here is that your method is very rigid. Kevin is trying to teach you how to make functions so that when a user calls them and chooses a random set of numbers, it will still calculate the same way. Your solution works, but only with those sets of numbers and can't be reused.
add_list: so...what I do is make a new function called add_list. It takes a list as the argument. It starts out with a "counter" of 0. then it uses a "for" loop to go through each number in the list and add it to the total. It then returns the total.
summarize: This one is similar. I set a total counter again. I loop through each number in list 2. Add them to the total. Now that the function is done using list2 variable...I convert it into a string using the .join and str function. Finally, I return my newly formatted list2string and total2 counter.
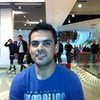
Andreas cormack
Python Web Development Techdegree Graduate 33,011 PointsHi Tristan
The Challenge asks you to create a function named add_list. This function should take a list as an argument ie [1,2,3] and return the total of [1,2,3] in this case 6. You will need a variable to hold the total value, you will also need a for loop. this is how you write a for loop
list = ["hello","Tristan"]
for word in list:
print(word)
# output would be
hello
Tristan
since we have 2 values in our list the loop goes round list twice printing word each time.
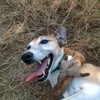
Cory Madden
7,120 PointsFirst of all, they're asking you to create functions not variables. So your first function you would create would be:
def add_list(num_list):
#since it says to use a for loop we'll do it that way
total = 0
for num in num_list:
total += num
return total
and then you'd have another function called summarize:
def summarize(num_list):
# do some work in here using the function add_list to format the string
return some_formatted_string
Tristan bradford
1,123 PointsTristan bradford
1,123 PointsThank you for the fast responses everyone. I get what they're going for now, and I'll give it an attempt in a few hours when I get home.