Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial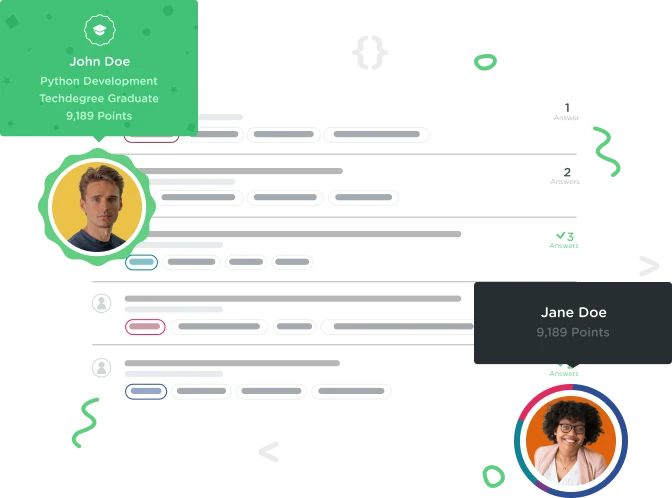

Aizah Sadiq
2,435 PointsI don't understand what this means
What does self.append(copy.copy(value))
mean. I understand that self.append adds whatever is added to the end of the list but what does the rest of it mean?
1 Answer
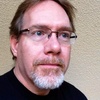
Chris Freeman
Treehouse Moderator 68,441 PointsSo you’ve got the part how
self.append(value)
would add the object value
to end of the list. It’s interesting that Kenneth chose the variable name “value” since this is closer to the truth. In Python, a list
contains only the reference ID value to each object in the list and not the actual object. The built-in function id
returns the unique id of an object (which is its location address in memory).
>>> item = [1, 2, 3]
>>> l = []
>>> for _ in range(3):
... l.append(item)
...
>>> for obj in l:
... print(id(obj))
...
4717438664
4717438664
4717438664
Each item in the list is the same item since they all have the same id.
Using copy
, a new replicated version is created separate from the original.
>>> for _ in range(3):
... l.append(copy.copy(item))
...
>>> for obj in l:
... print(id(obj))
...
4711909320
4711910600
4711915592
>>> l = []
Now, if the original object is changed, none of the copies will be affected.
Bonus: an immutable object is not copied by copy
. Since the an immutable object can’t change you don’t need more than one instance of it.
>>> obj = 'immutable object'
>>> obj == copy.copy(obj)
True
>>> id(obj)
4717502376
>>> id(copy.copy(obj))
4717502376
Post back if you need more help. Good luck!!!
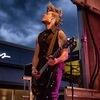
Nikolai Olekhnovitch
Front End Web Development Techdegree Graduate 25,617 PointsI am going through the Beginning Python track a second time and I'd like to shed some additional light on this subject.
The .copy() function is a function available through the copy library. Kenneth imports this when creating his FilledList class. Importing the library isn't enough to freely use its functions though, you need to reference the library when you call the function. The first part of the function is the library's name and the second part is the function's name. This is why you get copy.copy().
Here's another example:
If I wanted to use the .mean() function of a library called numpy, I would first import it in the script and have to write it out like this:
numpy.mean()
Kenneth eventually explains this in the Dates and Times in Python course.
EDIT: I was referring to .copy() as a method, but it is a function. I changed the text to reflect that.
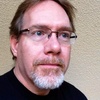
Chris Freeman
Treehouse Moderator 68,441 PointsNikolai Olekhnovitch, good comments. I might add the following additional usages
# import function to directly
from copy import copy
# now it can be used as copy()
# alias import import for convenience
import numpy as np
# use as
np.mean()
To clarify, copy()
is a function regardless of whether it is referenced as copy.copy()
or copy()
. A method refers to a function that is bound to a class definition
# import module
>>> import copy
>>> type(copy)
<class ‘module’>
# reference functions from module
>>> type(copy.copy)
<class ‘function’>
>>> type(copy.deepcopy)
<class ‘function’>
# import function for direct use
>>> from copy import copy
# This overwrites the module reference
# in local namespace
>>> type(copy)
<class ‘function’>
# other functions no longer available
# since copy now refers to function not module
>>> type(copy.deepcopy)
Traceback (most recent call last):
File “<stdin>”, line 1, in <module>
AttributeError: ‘function’ object has no attribute ‘deepcopy’
Nikolai Olekhnovitch
Front End Web Development Techdegree Graduate 25,617 PointsNikolai Olekhnovitch
Front End Web Development Techdegree Graduate 25,617 PointsChris Freeman thank you for the clarification! I had assumed that .copy() came from a class in the library.
Thank you also for including the alias import, that was new to me and that will certainly be handy!