Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial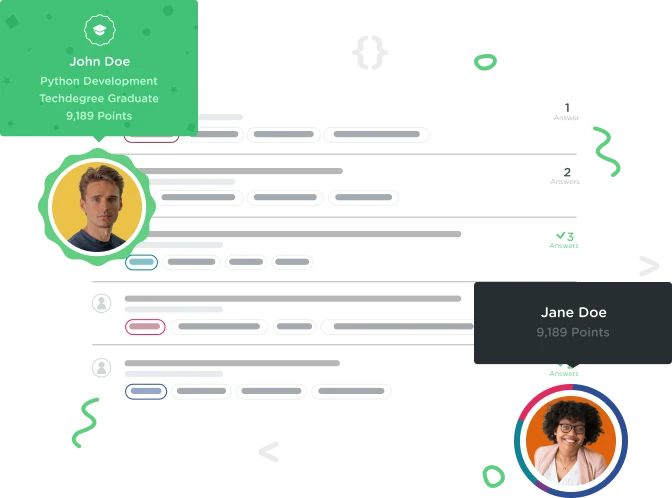

Rebecca St.Clair
5,088 PointsI don't understand what this question is even asking me to do.
The company you work for wants to send an email to its member base. They want the email to contain a complementary offer based on their membership level.
We've included a helper.php file that contain the connection to the database and give us a PDO object $db to use. This helper file also contains a function to send_offer. The send_offer function accepts the following arguments: $member_id, $email, $fullname, $level
In the current file, we've included the query to select the required items from the database. The results of the database query are stored in a PDOStatement object named $results. Loop through those results and pass them to the send_offer function.
Tip: we used this method in the last video
<?php
include "helper.php";
try {
$results = $db->query(
"SELECT member_id, email, fullname, level FROM members"
);
} catch (Exception $e) {
echo $e->getMessage();
}
//add code below this line
send_offer ($results->fetchAll(PDO::FETCH_ASSOC));
3 Answers
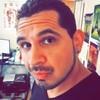
Daniel LittleThunder
6,975 Points<?php
include "helper.php";
try {
$results = $db->query(
"SELECT member_id, email, fullname, level FROM members"
);
} catch (Exception $e) {
echo $e->getMessage();
}
//add code below this line
$members = $results->fetchAll(PDO::FETCH_ASSOC);
foreach ($members as $member) {
send_offer($member['member_id'],
$member['email'],
$member['fullname'],
$member['level']
);
}
So, I'm answering this to help solidify it in my own mind, because I barely understood it as well. Forgive me if I'm not referencing everything correctly, I only started PHP a week or 2 ago. First, we need to fetchAll on the array items from the $results object and store the returned information in another variable for use in the foreach loop. In this case I chose $members as my array variable name, because the $results object is retrieving the information for members (plural) and I will eventually use the foreach loop to select each member (singular).
With the $members variable in place, I then used a foreach loop to store each iteration in the $member variable, and used the $member variable in the send_offer function for each of the relevant argument variables.
I hope this made sense. I don't quite know the entire terminology or how to use Markdown yet.

KRIS NIKOLAISEN
54,971 PointsIn your code you are sending an array. The challenge wants you to loop through the array (use foreach) and pass $member_id, $email, $fullname, $level to the send_offer function.

Rebecca St.Clair
5,088 PointsI tried that but it didn't work either. I tried two ways...
foreach ($results AS $result) { send_offer($member_id, $email, $fullname, $level); }
And ...
foreach ($results AS $result) { send_offer($result[$member_id], $result[$email], $result[$fullname], $result[$level]); }
but both times it keeps giving me this message. Bummer: Doesn't look like you are passing the correct values.
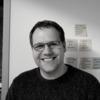
Jon McCallum
10,506 PointsThanks for your help, again with Treehouse, the question didn't quite explain itself, and the hint that the previous video held the solution had me stumped. I went with a more concise solution, but I'm not sure if it would be considered as best practice.
foreach ($results->fetchAll(PDO::FETCH_ASSOC) as $member) {
send_offer($member['member_id'],
$member['email'],
$member['fullname'],
$member['level']
);
}
Rebecca St.Clair
5,088 PointsRebecca St.Clair
5,088 PointsThank you, that really helped. Now that I see it that does make sense, I just could not understand what the question wanted.
and 2 weeks, wow, it's really impressive that you got this fare that fast.
Daniel LittleThunder
6,975 PointsDaniel LittleThunder
6,975 PointsI'm glad it helped. I've been doing anywhere from 10 to I think my highest was 60 video classes every day. I've also got a lot of programmer friends to lean on. And I have experience with JavaScript, so the c-based syntax and basic concepts like functions, variables, loops, and arrays aren't exactly new to me.