Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial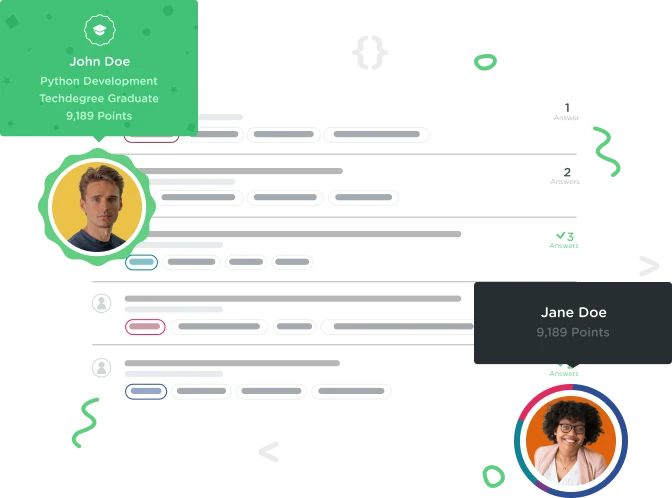
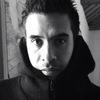
Jose Gutiérrez
9,370 PointsI don't understand what's happening! Can anyone help me, please?
This is my code: <?php
class Render { public $dimensions =array(); public function getDimensions($self){ return $self->dimensions; } public static function displayDimensions($size){ return $size[0] . " x " . $size[1]; } public static function detailsKitchen($room){ return "Kitchen Dimensions: " . Render::displayDimensions(getDimensions($room)); }
} $room = new Render(); $room->dimensions[] = (20); $room->dimensions[] = (10); ?>
This is the error:
PHP Fatal error: Uncaught Error: Call to undefined function getDimensions() in index.php:12 Stack trace: #0 6033c6f9-bc53-4bfa-9e1d-a403951652f5.php(176): Render::detailsKitchen(Object(Room)) #1 {main} thrown in index.php on line 12 Fatal error: Uncaught Error: Call to undefined function getDimensions() in index.php:12 Stack trace: #0 6033c6f9-bc53-4bfa-9e1d-a403951652f5.php(176): Render::detailsKitchen(Object(Room)) #1 {main} thrown in index.php on line 12
<?php
class Render {
public $dimensions =array();
public function getDimensions($self){
return $self->dimensions;
}
public static function displayDimensions($size){
return $size[0] . " x " . $size[1];
}
public static function detailsKitchen($room){
return "Kitchen Dimensions: " . Render::displayDimensions(getDimensions($room));
}
}
$room = new Render();
$room->dimensions[] = (20);
$room->dimensions[] = (10);
?>
1 Answer
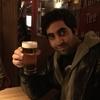
Ran ShemTov
14,148 PointsYou haven't encountered any request to create a "getDimensions" method anywhere, but instructed that the $room you will receive will already contain it (lets say programmer x has already created it in another php file). So I'd go deleting this method you have there, first.
Second: You could definitely use this challange passing example, where you see I did not use:
Render::displayDimensions(getDimensions($room));
I used "self"
self::displayDimensions($roomD);
Passing it a variable, in which I stored what comes back from the $room->getDimensions (that function which you shouldn't write) outcome.
<?php
class Render {
static function displayDimensions($size){
return $size[0].' x '.$size[1];
}
static function detailsKitchen($room) {
$roomD = $room->getDimensions();
return 'Kitchen Dimensions: '.self::displayDimensions($roomD);
}
}
?>
Last thing, no one asked to create a new instance of "Render" and get things working on it. Some programmers feel the urge to over complicate stuff and try writing extra lines. In this case, I'd suggest going over the "4 P's of programming" explained in some JavaScript courses by Andrew Chalkley (but I guess some other Treehouse teachers explains it as well in some other courses).
PRO TIP: Somtimes when we go over code out loud/read what we wrote like a book, things can get clearer on their own. Read about this absolutly-no-joke(!!) method and see if it gets you the results: http://www.rubberduckdebugging.com/ https://en.wikipedia.org/wiki/Rubber_duck_debugging
Hope this helps
Jose Gutiérrez
9,370 PointsJose Gutiérrez
9,370 PointsThanks a lot!! Now I got it! Next step: go and get my rubber duck! Thanks for the tip. it seems funny but I'm sure it's a good strategy. Sometimes I caught myself speaking loudly to the screen trying to get an answer... it's better if I have a rubber friend! Finally, I assume you omitted the "public" visibility in your method's declarations because the visibility by default is "public". Right?
Once again thanks!
Ran ShemTov
14,148 PointsRan ShemTov
14,148 Pointspublic is indeed default and doesn't need to be written