Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial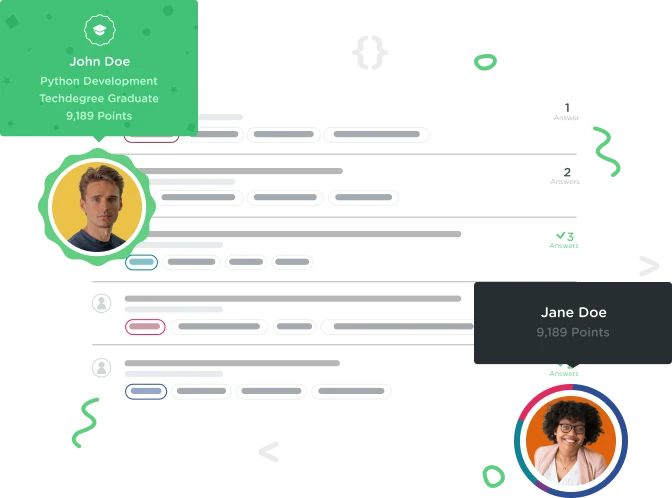

Thomas Katalenas
11,033 PointsI don't understand where I am going wrong here.
enum Coin: Int { case Penny = 1, Nickel = 5, Dime = 10, Quarter = 25
init(Money: Coin.rawValue){
switch Money {
case 1:
self = .Penny
case 5:
self = .Nickel
case 10:
self = .Dime
case 25:
self = .Quarter
default:
self = .Quarter
}
func num(anum: Int) -> Int {
return anum
}
func isGreater(currentValue: Coin, newValue: Coin) -> Bool {
return currentValue.rawValue > newValue.rawValue
}
isGreater.self
}
enum Coin: Int {
case Penny = 1, Nickel = 5, Dime = 10, Quarter = 25
init(Money: Coin.rawValue){
switch Money {
case 1:
self = .Penny
case 5:
self = .Nickel
case 10:
self = .Dime
case 25:
self = .Quarter
default:
self = .Quarter
}
func num(anum: Int) -> Int {
return anum
}
func isGreater(currentValue: Coin, newValue: Coin) -> Bool {
return currentValue.rawValue > newValue.rawValue
}
isGreater.self
}
3 Answers

Thomas Katalenas
11,033 Pointshey thanks alot, because of that little bit of help you gave me it made the whole thing work, just knowing that a correct answer was infront of me gave me the momentum I needed to fix my own problem. so basically the _ Money makes it so that you don't have to type print(Coin.init(Money:5)) which prints nickle. nice because then when I want to initialize an int I don't have to wory about Money descriptor, when I call a function later like num.

Keli'i Martin
8,227 PointsI think you might be overthinking the problem a little. All you need to do is initialize the enum with the value of Quarter. That can be accomplished as simply as this:
init() {
self = .Quarter
}
Hope this helps!

Thomas Katalenas
11,033 Pointsinitializers may only be declared within a type
So enum is the type, and then I had to make sure there was a type in side the initializer plus, I added _ so that I didn't have to type in Money all the time and then only numbers need to be typed.
Allthough I am wondering if I can put functions in side enums.
enum Coin: Int {
case Penny = 1, Nickel = 5, Dime = 10, Quarter = 25
init(){
self = .Quarter
}
init(_ Money: Int) { // _ : Intthis is great the code does actually work and all
let con: Int = Money.self // this is a code that works but I want to return defaul$
//let con = Coin.Penny so these two things return penny every time and Coin.Int m$
switch con{
//switch con.rawValue so these two things return penny every time
case 1:
self = .Penny
case 5:
self = .Nickel
case 10:
self = .Dime
case 25:
self = .Quarter
default:
self = .Quarter
}
}
func num(anum: Int) -> Int {
let anum: Int = 1
return anum
}
func isGreater(currentValue: Coin, newValue: Coin) -> Bool {
return currentValue.rawValue > newValue.rawValue
}
//isGreater.self
}
print(Coin.init(5))

Keli'i Martin
8,227 PointsWas all that extra code you put in just for extra practice? Because for the challenge, all you needed to do was add the initializer. Just adding
init() {
self = .Quarter
}
before the isGreater()
function was enough to pass the challenge.
Keli'i Martin
8,227 PointsKeli'i Martin
8,227 PointsNo problem! Glad to be of assistance.