Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial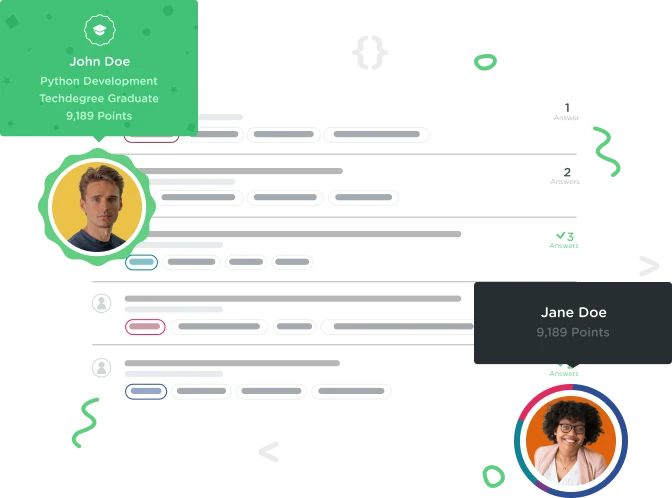
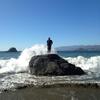
Joe Williams
4,014 PointsI don't understand why creating a function that subtracts a-b then sorts the numbers in the array.
Could someone explain this a little more clearly?
3 Answers

Ryan Boone
26,518 PointsI'm assuming you are referring to JavaScript's Array.prototype.sort() function. Array.sort() is only interested in the output of a function (what a-b equals). In the background, Array.sort() takes the arguments given it, then, one at a time, runs the compareFunction (in this case, a-b
) and decides what to do based on the answer given.
If the answer is less than 0, then a
moves to a lower index than b
. Greater than 0 moves b
to a lower index than a
. An answer of 0 means to leave the arguments where they are.
Think of it this way:
var array = [1,3,5,2];
// an array of 1, 3, 5 and 2
function compareFunc(a, b) {
return a-b;
};
// the comparison function we're using
array.sort(compareFunc);
// Calls this:
// 1-3 = -2, so 1 (a) is a lower index than 3 (b)
// 3-5 = -2, so 3 (a) is a lower index than 5 (b)
// 5-2 = 3, so 5 (a) is a higher index than 2 (b)
// The array now looks like this: [1,3,2,5]
// Array.sort() calls compareFunc() over and over again
// until every return is either a negative number or 0
// (or if it gets a different result from the same values
// in which case it returns undefined
Hope that makes sense.

Simon Tharby
2,951 PointsThis seems to be what is commonly called a 'bubble sort': http://en.wikipedia.org/wiki/Bubble_sort. The animation on the right of that wiki page clarifies the process, although I think the video example method starts at the other end of the list (array), and works backwards (from the right) in comparison to the wiki example, by swapping adjacent items if the left item of an adjacent pair is higher than the right item.
The video used here is not clear on how this method works, especially as it does not mention that this process is repeated along the array (or list) until no further changes are needed. For those with no knowledge of sorting, really it is just saying "this code will sort an array".
There are many other types of sorting algorithms, and choosing the right one can be important especially when ordering large lists (as the sorting process may take a long time, and the 'right' algorithm may reduce this time significantly).
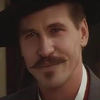
huckleberry
14,636 PointsThanks for that link. Much better answer than the constant "Oh it sorts by comparing two numbers and swapping the order based on comparison and I'm just regurgitating what the video said like a chimp mimicking its trainer but lulz I don't actually understand" responses I've been coming across.
That was the very issue I had with this ... WHY does it sort like that? How in the hell does TWO freakin' arguments sort a 200 element array or more???
But now, reading that bubble sort article and seeing the animation as well as reading the pseudo code, now I "get" not only what the anonymous compare function there is doing but what's going on in the behind-the-scene-code that is the sort
method.
And yes, considering how Hoskins' videos are more "technical" or rather more on the theory and academic side vs more applied theory like the new JS vids are, I'm surprised he didn't actually cover what the sort method actually does and I'm a little disappointed. Maybe some people don't care what's going on with their code and just want a snippet that they know "this does this" and that's it for them, but damn that's annoying
Cheers,
Huck -

Craig Neering
18,523 PointsThanks for the clarification, this didn't make sense to me either until it was more thoroughly explained!
David Lin
Full Stack JavaScript Techdegree Student 8,116 PointsDavid Lin
Full Stack JavaScript Techdegree Student 8,116 PointsThanks a bunch! Now I understand the logic behind array.sort(compareFunction) in this video!