Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial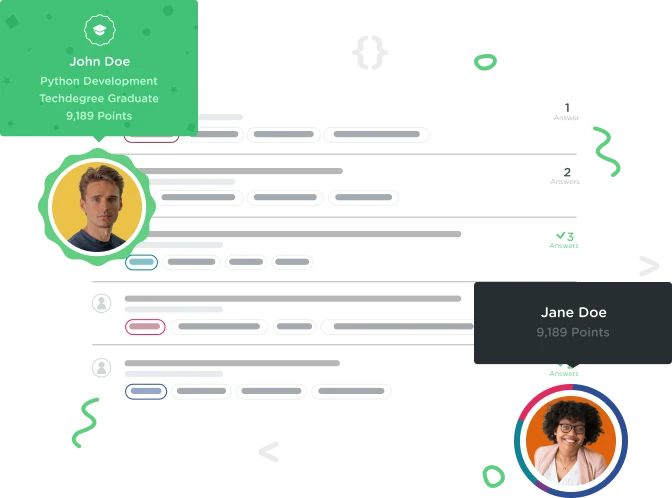
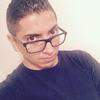
Eden Gomez
22,787 PointsI don't understand why this code don't work! Please I need help
Please help!!
var secret = prompt("What is the secret password?");
while (secret === "sesame") {
document.write("You know the secret password. Welcome." );
}
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title>JavaScript Loops</title>
</head>
<body>
<script src="app.js"></script>
</body>
</html>
4 Answers
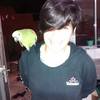
Juliana Demarque
14,502 PointsIn order to keeping it DRY (don't repeat yourself), I would just like to add a bit to Alexander's answer, changing the while loop to a do/while loop.
The difference is that you set the prompt method only once. It will execute the prompt method first thing, then, if the answer is wrong (!=="sesame"), it keeps on asking for the right password.
// There's no need to write this first line,
// because we will be setting the secret var inside the loop
// - don't need this anymore - var secret = prompt("What is the secret password?");
// Set the secret var to use the prompt method
do {
var secret = prompt("What is the secret password?");
} while (secret !== "sesame"); // ... and stay inside the loop until the answer is right
document.write("You know the secret password. Welcome." );
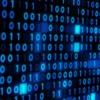
Alexander Davison
65,469 PointsThe goal of this challenge is to ask for the password until the person gets the password (which is sesame). Try this instead:
var secret = prompt("What is the secret password?");
// While the input isn't sesame (if it is already sesame then this loop will do nothing)...
while (secret !== "sesame") {
// ...then keep asking for the password
var secret = prompt("What is the secret password?");
}
document.write("You know the secret password. Welcome." );
Hope this helps! ~Alex
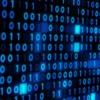
Alexander Davison
65,469 Pointswhen I answer people's questions, I like to keep my answers nice and simple. Although when I'm actually programming, I always be as DRY as possible :)
~Alex
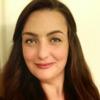
Jennifer Nordell
Treehouse TeacherHi there! While Alexander Davison provides a working solution, I'd like to provide an alternative that might be considered a little bit "DRY"er, if I may.
var secret = '';
while (secret !== "sesame") {
secret = prompt("What is the secret password?");
}
document.write("You know the secret password. Welcome." );
If we start secret equal to an empty string then it's by default not going to be equal to sesame. At this point the while loop will start and ask for the secret password until "sesame" is entered. It's not necessary to use var
to redeclare the variable through every iteration of the loop.
Hope this helps!
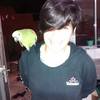
Juliana Demarque
14,502 PointsBetter yet! :)
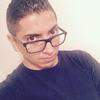
Eden Gomez
22,787 Pointsthank you so much guys!!