Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial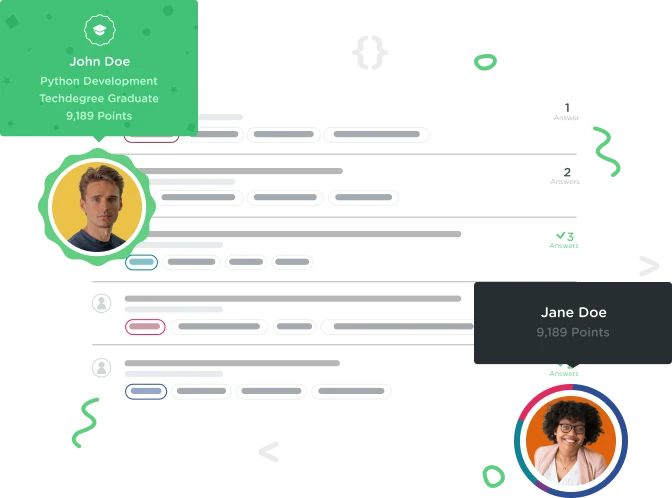

Niki Esfandiari
4,160 PointsI don't understand why this code won't work for the 'covers' question in the sets course
def covers(set1): ans = [] for course, topics in COURSES.items(): if len(set1.union(topics)) > 0: ans.append(course) return ans
COURSES = {
"Python Basics": {"Python", "functions", "variables",
"booleans", "integers", "floats",
"arrays", "strings", "exceptions",
"conditions", "input", "loops"},
"Java Basics": {"Java", "strings", "variables",
"input", "exceptions", "integers",
"booleans", "loops"},
"PHP Basics": {"PHP", "variables", "conditions",
"integers", "floats", "strings",
"booleans", "HTML"},
"Ruby Basics": {"Ruby", "strings", "floats",
"integers", "conditions",
"functions", "input"}
}
def covers(set1):
ans = []
for course, topics in COURSES.items():
if len(set1.union(topics)) > 0:
ans.append(course)
return ans
Ewerton Luna
Full Stack JavaScript Techdegree Graduate 24,031 PointsEwerton Luna
Full Stack JavaScript Techdegree Graduate 24,031 PointsYour code won't work because it will always return a list with all the courses in it.
Why? Take a look at this line of code:
This if statement will always return True. This topics variable will always have len > 1, since the key values in the COURSES dict are not empty. Even if you pass set1 as an empty set, len(set1.union(topics)) will still be > 1 for the reason I just mentioned. So your list nammed ans will be appended with all the courses.
I made a few changes on your code:
Remember to coerce ans into a set, otherwise the code will return a list with repeated items in it.
I just passed this challenge and my code was the following:
Just as a side note, try using a desktop IDE like PyCharm. Testing your code there will help you a lot.