Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial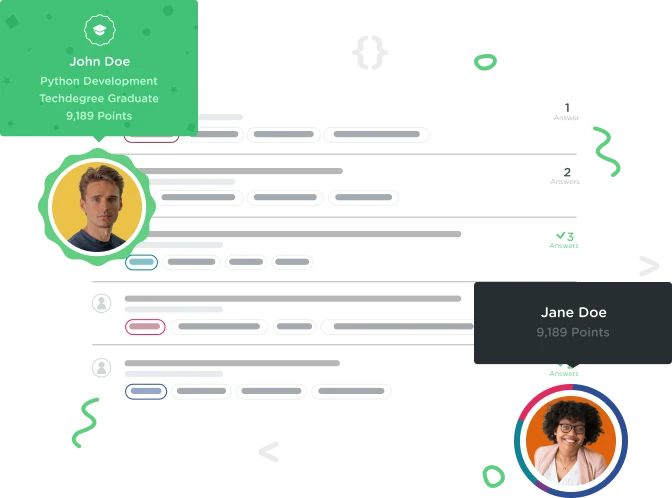
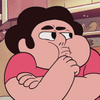
Ludwing Najera
4,596 PointsI don't understand why this doesn't work
It keeps giving me this message: "Make sure you throw a new IllegalArgumentException if the requested amount of laps would make the battery less than zero." The IF statement in the Drive class is correct, right?
class GoKart {
public static final int MAX_BARS = 8;
private String color;
private int barCount;
private int lapsDriven;
public GoKart(String color) {
this.color = color;
}
public String getColor() {
return color;
}
public void charge() {
barCount = MAX_BARS;
}
public boolean isBatteryEmpty() {
return barCount == 0;
}
public boolean isFullyCharged() {
return MAX_BARS == barCount;
}
public void drive() {
drive(1);
}
public void drive(int laps) {
lapsDriven += laps;
barCount -= laps;
if (laps >= MAX_BARS) {
throw new IllegalArgumentException("Not enough bars!");
}
}
}
1 Answer

andren
28,558 PointsThere are a couple of issues with the code:
- You are comparing
laps
againstMAX_BARS
instead ofbarCount
.MAX_BARS
contains the maximum bar value, not the current value,barCount
is the variable used to store the current value. - If I request 3 laps and there is 3 bars left then that is valid request, so
laps
being equal tobarCount
should not throw an exception. Meaning that you should be checking iflaps
is greater thanbarCount
, not greater or equal to. - Since your exception is meant to stop a lap from occurring it should be placed at the top of the method, not at the bottom.
If you fix those three issues like this:
public void drive(int laps) {
if (laps > barCount) {
throw new IllegalArgumentException("Not enough bars!");
}
lapsDriven += laps;
barCount -= laps;
}
Then your code will pass.