Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial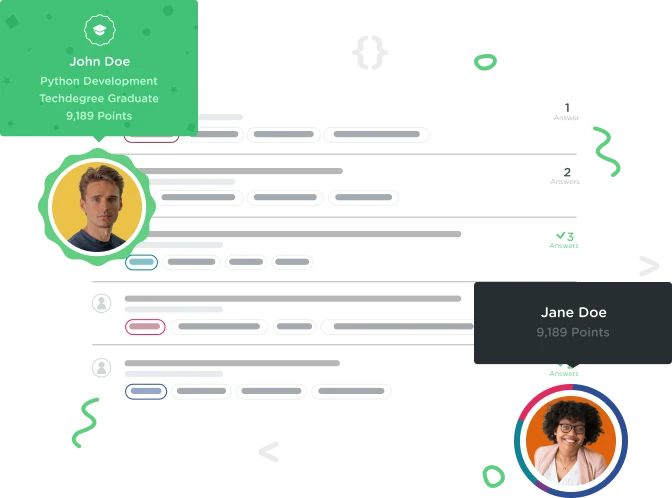

Esmobrander Ozpedelamo
9,136 PointsI don't understand why this is an instance of TodoListItem.
in the quiz there's a question that says: Given the following code what is the best way to describe task?
The right answer is It's an instance of TodoListItem, but i don't understand why to me its a constructor function.
function TodoListItem(description) {
this.description = description;
this.isDone = false;
this.toString = function() {
return this.description;
}
this.markAsComplete = function() {
this.isDone = true;
}
}
var task = new TodoListItem("Do the laundry!");
4 Answers

Dan Oswalt
23,438 PointsThink of a constructor function as a factory that creates objects. This factory produces ToDoListItems. When you call the constructor function with the 'new' keyword, it produces a unique ToDoListItem, and it is stored as task. This unique product is known as an instance.
'Task' is not a constructor function. It is not the factory, it's the product.
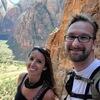
Jeremy Castanza
12,081 PointsI thought that I might try to weigh in on this. I think that thing tripping everyone up is probably the "this" key word. To conceptualize the idea of a constructor function, I tried using static code so that it was more relateable.
// CONSTRUCTOR FUNCTION (TEMPLATE) FOR ZOO ANIMALS
function Animal(type, age, name, noise) {
// sample output of the object that this constructor will build
/* this (instance #1 animal) = {
type: "Cat",
age: 5,
name: "Cuddles",
sound: "Meow",
makeNoise: function() {alert("Meow");}
} */
/* this (instance #2 animal) = {
type: "Elephant",
age: 27,
name: "Biggie",
sound: "Trumpet",
makeNoise: function() {alert("Trumpet");}
} */
/* template based on constructor */
this.type = type;
this.age = age;
this.name = name;
this.noise = noise;
this.makeNoise = function() {alert(this.noise)};
}
// FOUR INSTANCES of the Constructor Animal
var cat = new Animal("Cat", 5, "Cuddles", "Meow");
var elephant = new Animal("Elephant", 27, "Biggie", ");
var tiger = new Animal("Cat", 8, "Tony", "Roar", "Trumpet");
var zebra = new Animal("Zebra", 5, "Stripes", "Neigh");
Andrew Chalkley, as time permits, can you and the Treehouse crew consider a rework of the JavaScript constructor video? I think it would help to have a breakdown of the constructor concept without using "this", then introducing both the constructor and "this" concepts later on in the video after there's a solid foundation for the purpose behind a constructor and how it actually creates separate object instances using hard coded tangible properties and methods. The code above is meant to assist with some brainstorming around that idea. It's still pretty rough, but hopefully you get what I'm saying. Thanks!

Esmobrander Ozpedelamo
9,136 Pointsim still SOOOO confused...
you said: 'Task' is not a constructor function. It is not the factory, it's the product.
witch means that var balrog = new Monster("Captain");
its not the factory either.
that puts me back where i started, both pieces of code are the same ( not sure what they are /: )
I break that code in two parts.
FIRST:
function TodoListItem(description) {
this.description = description;
this.isDone = false;
this.toString = function() {
return this.description;
}
this.markAsComplete = function() {
this.isDone = true;
}
}
TWO:
var task = new TodoListItem("Do the laundry!");
when i see both pieces of code the one with the monsters and the one with the TodoListItem they're pretty much similar i mean to me they're the same is essence... keep wondering whats the real difference...
Andrew Chalkley Your assistance would be more than appreciated in this matter.
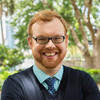
Andrew Chalkley
Treehouse Guest Teachervar task1 = new TodoListItem("Do the laundry!");
var task2 = new TodoListItem("Feed the chickens!");
task1
and task2
are the product of the constructor functions not the constructor function itself. The result of a constructor function with the new
keyword is an instance.
If you do the following code:
task1 instanceof TodoListItem;
Resolves to true
.
Variable names can be anything and understanding that they are an instance of something means you know you can call methods and access properties for that particular type, in this case it's a TodoListItem
.

Esmobrander Ozpedelamo
9,136 PointsAndrew Chalkley so in this case:
function Monster(rank) {
this.rank = rank;
this.health = 100;
this.takeHit = function() {
this.health--;
}
this.isDead = function() {
return this.health <= 0;
}
}
var monster = new Monster("Captain");
var monster its an instance of Monster(), right?
I think that all my confusion came from monster - Monster I think that you guys should change that example from the quiz ( or was it on purpose? ).
I had the concept pretty much clear before I got the quiz and I think that quiz got me confused.
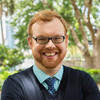
Andrew Chalkley
Treehouse Guest Teachermonster
is an instance of Monster
yes.
Remember variables are case sensitive. So, monster
!== Monster
, like firstName
and firstname
are different variables.
I think that all my confusion came from monster - Monster I think that you guys should change that example from the quiz ( or was it on purpose? ).
It wasn't on purpose, would monster1
be enough difference though?

Devjyoti Ghosh
2,422 PointsAndrew Chalkley can you explain in the above example how is
var balrog = new Monster("Captain");
//different from
var monster = new Monster("Captain");
Esmobrander Ozpedelamo
9,136 PointsEsmobrander Ozpedelamo
9,136 Pointsthen what's the difference between that and this:
Dan Oswalt
23,438 PointsDan Oswalt
23,438 PointsWhat's the difference between monster and Monster? In your example, monster is an instance of the Monster class. One is a constructor function, the other is a monster object.
Presumably, if you are creating a reusable function to construct monsters, you're going create more than one, that's the whole point. So you probably would want to name it something other than 'monster'.
balrog and orc and flyingMonkey are instances. Monster() is just a factory for instances of monsters.
Dan Oswalt
23,438 PointsDan Oswalt
23,438 Pointsyou can't make new monsters with these instances. They are objects. If you console.log(monster), you see an object. console.log(Monster) and you see a function.