Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial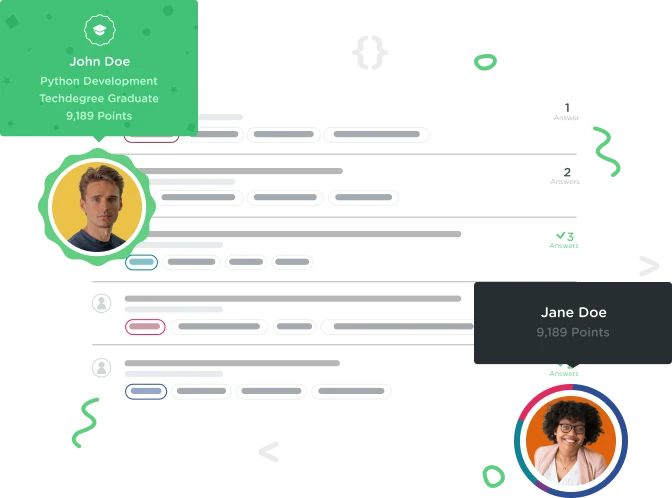

nicolaspeterson
8,569 PointsI don't understand why we define variables for is_fizz and is_buzz.
Can someone please explain this part? The TODO asks us to define variables for is_fizz and is_buzz that stores a Boolean value of the condition. Also, why do we need the modulo operator?
This challenge was very confusing and difficult.
3 Answers
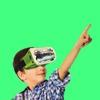
John Hill
Front End Web Development Techdegree Graduate 35,236 PointsHe uses the variables and booleans to keep the code cleaner. You could put everything in an if-statement like this...
if number % 3 == 0 and number % 5 != 0:
print("Your number", number, "is a Fizz number")
elif number % 5 == 0 and number % 3 != 0:
print("Your number", number, "is a Buzz number")
elif number % 3 == 0 and number % 5 == 0:
print("Your number", number, "is a FizzBuzz number")
else:
print("Your number", number, "is neither a fizzy or a buzzy number.")
...but you'd be repeating yourself a lot. I'd guess his way runs a bit faster too since it performs the math calculations once and then compares the variables in the if-statment instead of having an if-statement full of math. That might be negligible here, but could be a significant performance boost in a large program. (Can an expert confirm?)
He's also showing us that variables can be created conditionally.
is_fizz = number % 3 == 0
is_buzz = number % 5 == 0
The number will be assigned to is_fizz only if the remainder after dividing by 3 is 0; it will be assigned to is_buzz only if the remainder after dividing by 5 is 0. Then he creates his if-statement using these variables.
Also, why do we need the modulo operator?
The modulo gives the remainder. If the remainder after division is 0, that lets you know the number is evenly divisible. For example: 9 % 3 = 3.0 and 10 % 5 = 2.0. Since these numbers have 0 as the remainder, you know that 9 is divisible by 3 and 10 is divisible by 5. If your number is 15, the remainder will be 0 after dividing by either 3 or 5.

Josh Keenan
20,315 PointsSo these two variables will store the output from
is_fizz = number % 3 == 0
is_buzz = number % 5 == 0
These are either going to be True, or False.
is_fizz
will evaluate, when the number is divided by 3, is there no remainder, if there is no remainder, I am True, otherwise I am False. The modulo operator divides and keeps the remainder, so if number is 6, is_fizz is true, is_buzz is false, 15 would give True and True, 16 would give False, False.
Feel free to ask any other questions
Also I see you have lots of questions, I'd advise you slow down with the content and don't just view it, go over it again and again as you need to learn and absorb this information, it takes time and effort. You got this!

Roman Schenk
5,207 PointsYou don't need to understand why they defined those variables as there is no need for them. They did it probably to make you code more and use more of the things you learned. number_int = int(number)
output = "" if (number_int % 3) == 0: output += "Fizz" if (number_int % 5) == 0: output += "Buzz" if not bool(output): output = "is neither a fizzy or a buzzy number."
print(output)
This would work as well. All you do with the variables is to store the result, where as here it get's lost.
Mathias Langner
4,156 PointsMathias Langner
4,156 PointsThank you very much for this nice explanation. Also questioned myself why we define variables, because it's also possible to do everything in the if-statement. But yeah, clean and non-repetitive makes sense !