Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial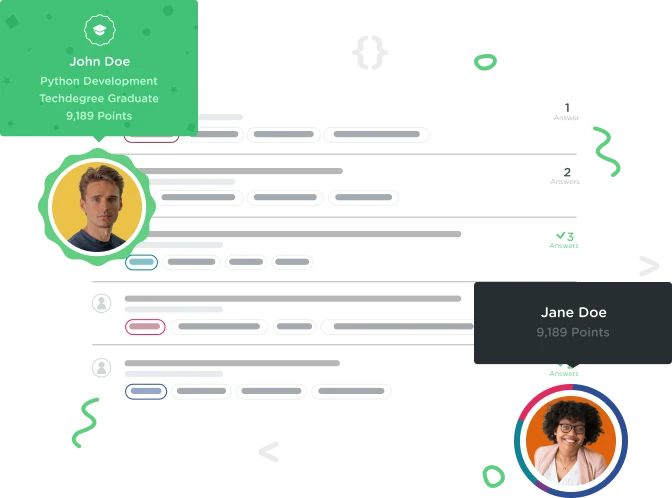
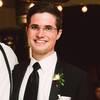
Zachary Luke
Front End Web Development Techdegree Graduate 17,646 PointsI feel I'm getting close to solving, but I keep getting an undefined error.
I know I've got the .getElementById selector correct, and I've got the children selector in the correct place, but it keeps saying there's an "undefined" error in the listItems[i].style.color portion of the code.
How can I solve this?
const listItems = document.getElementById('rainbow');
var colors = ["#C2272D", "#F8931F", "#FFFF01", "#009245", "#0193D9", "#0C04ED", "#612F90"];
for(let i = 0; i < rainbow.children.length; i ++) {
listItems[i].style.color = colors[i];
}
<!DOCTYPE html>
<html>
<head>
<title>Rainbow!</title>
</head>
<body>
<ul id="rainbow">
<li>This should be red</li>
<li>This should be orange</li>
<li>This should be yellow</li>
<li>This should be green</li>
<li>This should be blue</li>
<li>This should be indigo</li>
<li>This should be violet</li>
</ul>
<script src="js/app.js"></script>
</body>
</html>
1 Answer

Marcus Mayorga
11,312 PointsI think I see what you were trying to do here but the way the for loop was set up originally was fine because it cycled through the array of colors. Focus on the listItems constant by referring to a "collection."
I don't think you can use getElementById in this case. This got the job done for me:
const listItems = document.querySelectorAll('#rainbow li');
var colors = ["#C2272D", "#F8931F", "#FFFF01", "#009245", "#0193D9", "#0C04ED", "#612F90"];
for(var i = 0; i < colors.length; i ++) {
listItems[i].style.color = colors[i];
}
Marcus Mayorga
11,312 PointsMarcus Mayorga
11,312 PointsActually, your for loop would work as well. Just change the listItems const.