Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial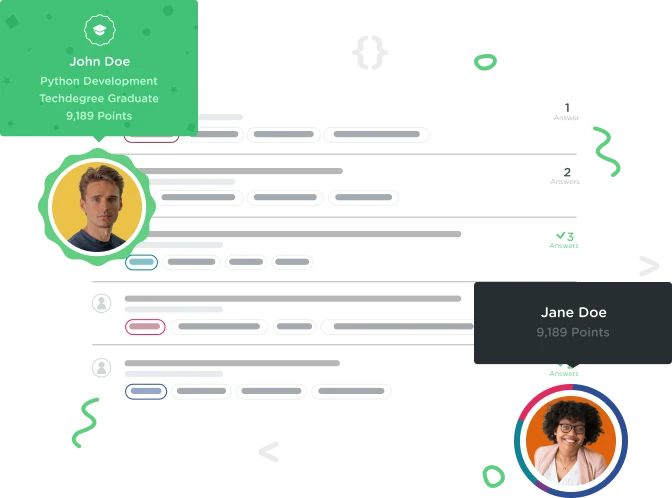

James Kane
Front End Web Development Techdegree Student 3,070 PointsI feel like I made this more complicated than it needed to be but it was the only solution I could think of, help plz
I think this would work as a method but obviously its not what Craig is looking for, any help much appreciated :)
public class Order {
private String itemName;
private int priceInCents;
private String discountCode;
public Order(String itemName, int priceInCents) {
this.itemName = itemName;
this.priceInCents = priceInCents;
}
private String normalizeDiscountCode(String discountCode) {
return discountCode.toUpperCase();
for (int i = 0; i < discountCode.length(); i++) {
char validationCheck = discountCode.charAt(i);
if (validationCheck != "[A-Z]") || (validationCheck != "[$]") {
throw new IllegalArgumentException("Invalid discount code");
}
}
}
}
// snipped for brevity
public void applyDiscountCode(String discountCode) {
this.discountCode = normalizeDiscountCode(discountCode);
}
}
1 Answer
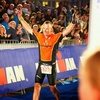
Steve Hunter
57,712 PointsHi James,
Firstly, you are immediately returning out of the normalizeDiscountCode
method so most of your code won't be reached. Move the return to the bottom of the method; it should be the last line.
After doing that you get a load of errors with your comparisons. Let's break down a different way of iterating over a string. We can use a method called toCharArray()
which converts your string to an array of chars. We can then use a for:in loop to pull out each character in turn. It would look like this:
for(char letter : discountCode.toCharArray()){
// do some stuff with each letter
}
So, now we have each letter isolated in turn and stored in the local variable, letter
. We want, first, to see if it is a letter. There's a method, it's a class method, on the Character
class called isLetter()
. No prizes for guessing what it does! It returns true
or false
depending on what is passed in, i.e. is the parameter a letter. It's a class method so we call it on Character
, chained with dot notation, and pass in the letter
variable like this: Character.isLetter(letter)
.
We also need to test if letter
is/isn't a dollar sign. We need to consider the logic carefully, here. We want to throw an exception if
the character is NOT a letter AND is NOT a dollar sign. So we need to negate the isLetter
test with a preceding !
, and also test, with !=
if the character is not '$'. That needs joining with an AND, &&
. If both those conditions are met, hence the &&
, throw the exception.
That could look like:
for(char letter : discountCode.toCharArray()){
if(!Character.isLetter(letter) && letter != '$'){
// throw the exception & message here
}
}
That's it - if the code execution has made it this far, return the uppercase version of discountCode
.
I'll leave you to put all that together - let me know how you get on!
Steve.
Steve Hunter
57,712 PointsSteve Hunter
57,712 PointsP.S. Make sure you return the uppercase
discountCode
outside the loop and before the end of the method.James Kane
Front End Web Development Techdegree Student 3,070 PointsJames Kane
Front End Web Development Techdegree Student 3,070 PointsAwesome Steve thanks a lot I understand now :)
Steve Hunter
57,712 PointsSteve Hunter
57,712 Points