Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial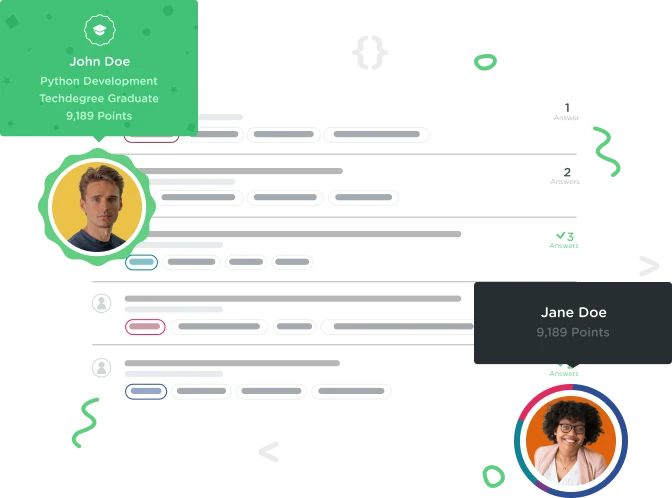

Brandon Gibbs
9,636 PointsI feel like I might have missed something here.... section challenge.
Ok.... So, I don't know if I missed something covered in the course or if I'm not 100% understanding what they are asking us to do in this challenge.
The task is: Almost done! Now, replace the value of "Treehouse" in greeting_list with the first item in your name_list variable.
My code to this point, from task 1 and 2, is below. All of it checks out... I'm just not sure where to go from there with what they are asking.
full_name = "Brandon Gibbs"
name_list = full_name.split()
greeting_list = "Hi, I'm Treehouse".split()
4 Answers
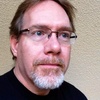
Chris Freeman
Treehouse Moderator 68,423 PointsHere is a basic flow through the challenge. Everything is based off of material covered in the videos:
# Task 1 of 4
# Create a variable named 'full_name' that holds your full name.
# Make another variable named 'name_list' that holds your full
# name in list form, split on the spaces. Don't create name_list
# manually, use split!
full_name = "Chris Freeman"
name_list = full_name.split()
# Task 2 of 4
# Create a variable named 'greeting_list' that uses the string
# "Hi, I'm Treehouse" to create a list by splitting on the spaces.
greeting_list = "Hi, I'm Treehouse".split()
# Task 3 of 4
# Almost done! Now, replace the value of "Treehouse" in
# greeting_list with the first item in your name_list variable.
greeting_list[2] = name_list[0]
# Task 4 of 4
# Great! Finally, make a variable named 'greeting' where you join
# 'greeting_list' back into a string, separated by spaces. You
# should end up with "Hi, I'm **<your first name>**" as the value
# of greeting.
greeting = ' '.join(greeting_list)

Brandon Gibbs
9,636 PointsOH... OK!!! Now THAT I recognize from the videos now that I see it! This is MUCH more simple! This makes MUCH more sense to me at this stage.
This takes the 2 place in the variable for greeting_list and replaces it with the 0 spot (my first name). Thank you. I can't believe I got stuck on this. I took a break earlier... now it might be time to call it a night. lol
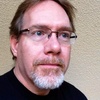
Chris Freeman
Treehouse Moderator 68,423 PointsIf you're in a situation where you do not know the index of the item you want to replace, you can get it using the .index()
method:
>>> name = "Zaphod Beeblebrox"
>>> name_list = name.split()
>>> greeting_list = "Hi, I'm Treehouse".split()
>>> idx = greeting_list.index('Treehouse')
>>> greeting_list[idx] = name_list[0]
>>> greeting = ' '.join(greeting_list)
>>> greeting
"Hi, I'm Zaphod"

Brandon Gibbs
9,636 PointsThat is actually really cool! I'm going to have to remember that one. I feel like that would come in handy, big time!
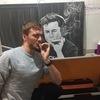
Tobias Mahnert
89,414 PointsBut if you have to send multiply request, u repeat yourself with the basic functionality especially if you don't know the index of both lists. My code is more efficient for complex structures but overload for a simple code challenge.
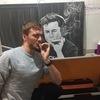
Tobias Mahnert
89,414 PointsHi Brendon,
kenneth is one of the most complicate teachers at treehouse. He often expects you to do some additional research to his challenges which is understandable if you want to work in IT industry where its all about creativity and stuff. anyway, this code passes. If you want to know why, let me know.
full_name = "Tobias Mahnert"
name_list = list(full_name.split())
greeting_list = list("Hi, I'm Treehouse".split())
def replace(l, X, Y):
for i,v in enumerate(l):
if v == X:
l.pop(i)
l.insert(i, Y)
l = greeting_list
replace(l, "Treehouse", "Tobias")
greeting = ' '.join(greeting_list)
cheers toby

Brandon Gibbs
9,636 PointsWOW... yeah almost none of that was covered except the last line of code.
I would love to know why / how that works.
I am 100% on board with doing the extra research.... I just figure that the test would kinda coincide with the courses...and at least send you on your way to do more research if it's going to be required in the challenge. Otherwise it severely breaks the flow. I guess that's all part of it though.
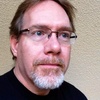
Chris Freeman
Treehouse Moderator 68,423 PointsThis is the most complicated solutions I've seen for this challenge. It most cases, if it is getting complicated there is likely a simpler way. See my posted answer.

Brandon Gibbs
9,636 PointsWhile I appreciate the help, and that did pass.... I had to go with the other answer. It was much more simple and was in line with what has been taught so far.
Thank you very much. I will, of course, be looking forward to learning more and doing my own research so that I have the skills to do more than repeat what they're teaching... but to actually create.
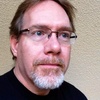
Chris Freeman
Treehouse Moderator 68,423 PointsApologies. I don't mean to imply your code is wrong or un-DRY. I applaud your creativity in making the replace
function. I have often solved a programming task with a home-brew solution, then presented to a peer who said "Why didn't you just use package X method Y." {face-palm}. It's a road all programmers travel.
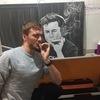
Tobias Mahnert
89,414 PointsChris answer is better for the challenge, my answer is better for long complex lists where you don't know the index, or too lazy to look it up

Brandon Gibbs
9,636 PointsYeah, I can see how finding the index could be a pain in longer lists.

Brandon Gibbs
9,636 PointsYeah, I can see how finding the index could be a pain in longer lists.
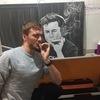
Tobias Mahnert
89,414 Pointsyea, i totally understand what you mean. I had my troubles myself, especially when you start i think there are better ways to approach this kind of "you have to do your part" thing. This is kinda he trows you in a hole with a piece of wool and expects you to build a rope from it, without manual, lol. anyway. So what I did is creating a function (thats the def part) and in this function is named replace. If you don't know what a function is, it is some kind of tool that lets you perform a certain task. Its like function == you build your own tools. This function I use it to replace a word with another word in a list variable and declare it to the greeting variable. i call (use the tool) the function by typing replace. the first argument is l which is the list i want to work with. the second argument is the Word I want to replace, in this case treehouse and the third argument is the new word that I gonna put in. In this case, my name Tobias.
Any more questions?
Brandon Gibbs
9,636 PointsBrandon Gibbs
9,636 PointsEven if it's not the answer... just a nudge in the direction of what I am supposed to do with it, or where in the video I missed what needs to be done here... I would be super grateful.