Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial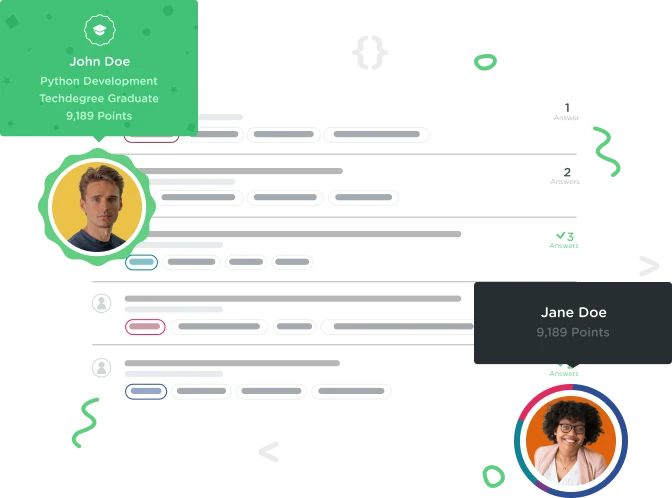

Bryn Walker
3,404 PointsI feel like I've misunderstood the challenge...
I'm not 100% sure I'm writing the write code to answer the question. As far as I understand, the challenge is asking me to write a method that will take self.player (in this case, it equals 1), and depending on the value of self.player, will increment that player's score by one, then return both the scores.
But I've tried dozens of different variations on this code, and all I get is 'Bummer! Try again!'. The code works as intended, as I've run it in workspaces, no problem.
The code -
class Game:
def __init__(self, **kwargs):
self.current_score = [0, 0]
self.player = input("Input 1 or 2")
for key, value in kwargs.items():
setattr(self, key, value)
def score(self):
if self.player == 1:
self.current_score[0] = self.current_score[0] + 1
if self.player == 2:
self.current_score[1] = self.current_score[1] + 1
return self.current_score
Apologies if I screwed up my code formatting ;)
2 Answers
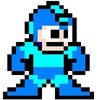
Robert Richey
Courses Plus Student 16,352 PointsHi Bryn,
Your code can work with a few minor tweaks.
- All class methods should have at least one argument 'self' (which you have), but the challenge also says that score takes a player argument as well
- The Game class has no attribute
player
so invokingself.player
doesn't work. Just use the passed-in player - Finally, the challenge is not expecting a return value, so you can leave this off
With these changes, I believe your code should pass - I did test it first to make this claim. Let me know if you continue to have any issues.
Best Regards

shezazr
8,275 PointsThe question says that the score method takes an ARGUEMENT either player 1 or player 2. it then increases that player's score by 1 in the current_score list
Robert Richey
Courses Plus Student 16,352 PointsRobert Richey
Courses Plus Student 16,352 PointsAlso, post if you solve this. Then I'll show you a one line solution - I promise this isn't gloating. As I continue learning to program it always helps to see how others approach and solve a problem. Then you can decide for yourself whether to adopt new patterns or not.
Cheers
Bryn Walker
3,404 PointsBryn Walker
3,404 PointsWow, thanks a lot Richard! About 10 seconds of rearranging code based on your suggestions and it worked. I'd tried various combinations of arguments etc. but none of them had worked until now.
I'm curious to know how you solved it though, I took a look at it and couldn't quite figure out how to do it in one line.
Robert Richey
Courses Plus Student 16,352 PointsRobert Richey
Courses Plus Student 16,352 PointsHey that's great, glad I could help! Here is my one line solution, enjoy :)